使用vue3加ts编写登录界面
时间: 2024-09-23 14:13:32 浏览: 87
在 Vue 3 中使用 TypeScript 编写登录界面,你可以按照以下步骤进行:
1. **安装依赖**:
- 首先确保已安装 Vue CLI 和 Vue 3 的 TypeScript 插件。运行 `npm install -D @vue/cli@next typescript` 或者使用 yarn 安装。
2. **配置项目**:
- 在 `.vuepress/config.js` 或者 `.babelrc` 中添加 TypeScript 支持,如设置 `"module": "esnext", "target": "esnext"`, 并启用插件如 `@vue/cli-plugin-typescript`。
3. **创建组件**:
- 创建一个名为 `Login.vue` 的文件,开始定义登录组件结构:
```html
<template>
<div class="login-form">
<input v-model="username" placeholder="用户名" />
<input type="password" v-model="password" placeholder="密码" />
<button @click="handleSubmit">登录</button>
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
data() {
return {
username: '',
password: '',
};
},
methods: {
handleSubmit() {
// 这里可以添加登录验证逻辑
},
},
});
</script>
```
4. **类型注解**:
- 对数据属性和方法进行类型注解,增强代码的可读性和安全性:
```typescript
data() {
return {
username: string,
password: string,
};
},
methods: {
handleSubmit(): void {
//...
},
},
```
5. **样式管理**:
- 可以选择使用 CSS-in-JS(例如 styled-components)或预处理器(例如 Less/Sass/SCSS)配合 TypeScript。
6. **导入并使用组件**:
- 在其他地方导入 Login 组件,并作为 props 或者直接在模板中使用。
```typescript
<template>
<div>
<Login @submit="handleLogin"></Login>
</div>
</template>
<script setup lang="ts">
import Login from '@/components/Login.vue';
const handleLogin = (event: Event) => {
event.preventDefault(); // 阻止表单默认提交
// 进行登录逻辑处理
};
</script>
```
阅读全文
相关推荐
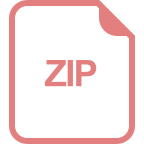
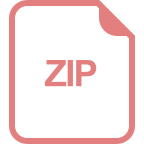
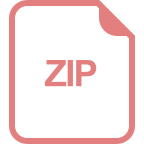
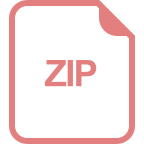
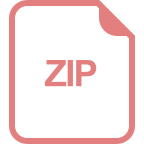
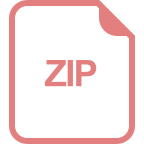
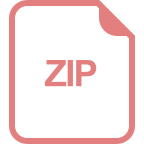
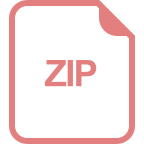
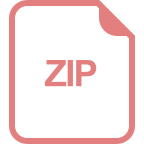
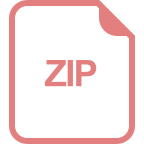
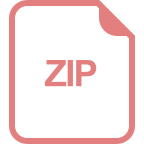
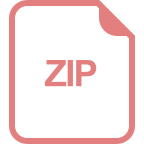
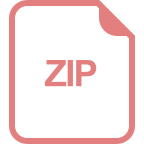
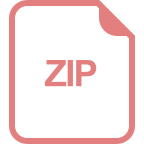
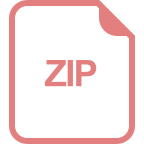
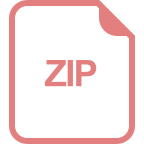
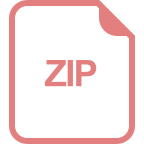
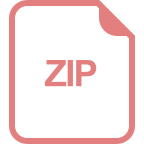