yolov5数据集分析代码
时间: 2023-10-03 13:10:48 浏览: 38
以下是一个基于YOLOv5的数据集分析代码示例:
```python
import os
import matplotlib.pyplot as plt
import json
def analyze_dataset(data_dir):
annotations_dir = os.path.join(data_dir, "annotations")
images_dir = os.path.join(data_dir, "images")
# 统计每个类别的物体数量
class_counts = {}
# 统计每张图像中物体的平均数量
object_counts = []
# 统计图像的宽度和高度分布
image_widths = []
image_heights = []
# 加载标注文件
annotation_files = os.listdir(annotations_dir)
for anno_file in annotation_files:
with open(os.path.join(annotations_dir, anno_file), 'r') as f:
annotation_data = json.load(f)
# 统计物体数量
objects = annotation_data['objects']
object_counts.append(len(objects))
# 统计每个类别的物体数量
for obj in objects:
obj_label = obj['label']
if obj_label not in class_counts:
class_counts[obj_label] = 1
else:
class_counts[obj_label] += 1
# 加载图像文件并统计宽度和高度
image_file = os.path.join(images_dir, anno_file.replace(".json", ".jpg"))
image = plt.imread(image_file)
image_heights.append(image.shape[0])
image_widths.append(image.shape[1])
# 绘制物体数量直方图
plt.figure(figsize=(10, 5))
plt.bar(class_counts.keys(), class_counts.values())
plt.xlabel('Class')
plt.ylabel('Count')
plt.title('Object Count by Class')
plt.xticks(rotation=45)
plt.show()
# 绘制物体平均数量直方图
plt.figure(figsize=(10, 5))
plt.hist(object_counts, bins=range(0, max(object_counts)+1))
plt.xlabel('Object Count')
plt.ylabel('Image Count')
plt.title('Object Count per Image')
plt.show()
# 绘制图像宽度和高度分布图
plt.figure(figsize=(10, 5))
plt.hist(image_widths, bins='auto', alpha=0.5, color='r', label='Width')
plt.hist(image_heights, bins='auto', alpha=0.5, color='b', label='Height')
plt.xlabel('Size')
plt.ylabel('Count')
plt.title('Image Size Distribution')
plt.legend(loc='upper right')
plt.show()
# 使用示例
data_dir = '/path/to/dataset'
analyze_dataset(data_dir)
```
这段代码会分析YOLOv5数据集的标注文件和图像文件,统计每个类别的物体数量、每张图像中物体的平均数量以及图像的宽度和高度分布。最后,会绘制出物体数量直方图、物体平均数量直方图和图像宽度和高度分布图。你需要将代码中的`/path/to/dataset`替换为你实际的数据集路径。
相关推荐
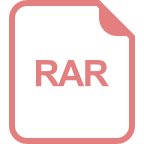
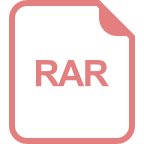
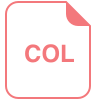
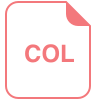
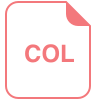
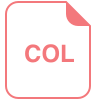
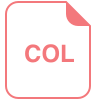









