3、 实现一个倒计时时钟,创建子线实现计时功能,使用异步消息机制将计时结果显示在界面上,如图,在文本框中输入数字(使用Number文本框),点击开始按钮后,下方文本框的数字每秒减1,点击停止即停止倒计时
时间: 2023-12-07 17:04:32 浏览: 200
这是一个基本的倒计时时钟的实现,使用了子线程实现倒计时,并通过消息机制更新UI界面。
```python
import tkinter as tk
import threading
class CountdownTimer:
def __init__(self, master):
self.master = master
self.countdown = tk.IntVar()
self.text_label = tk.Label(self.master, textvariable=self.countdown, font=('Arial', 36))
self.text_label.pack(pady=20)
self.input_label = tk.Label(self.master, text='Enter countdown time:', font=('Arial', 18))
self.input_label.pack(pady=10)
self.input_box = tk.Entry(self.master, font=('Arial', 18))
self.input_box.pack(pady=10)
self.start_button = tk.Button(self.master, text='Start', font=('Arial', 18), command=self.start_countdown)
self.start_button.pack(pady=10)
self.stop_button = tk.Button(self.master, text='Stop', font=('Arial', 18), command=self.stop_countdown)
self.stop_button.pack(pady=10)
self.timer_thread = None
self.is_running = False
def start_countdown(self):
if self.is_running:
return
try:
self.countdown.set(int(self.input_box.get()))
except ValueError:
self.countdown.set(0)
if self.countdown.get() > 0:
self.is_running = True
self.timer_thread = threading.Thread(target=self.countdown_timer)
self.timer_thread.start()
def countdown_timer(self):
while self.countdown.get() > 0:
self.countdown.set(self.countdown.get() - 1)
self.master.after(1000, self.update_text)
time.sleep(1)
self.is_running = False
def update_text(self):
self.text_label.config(text=self.countdown.get())
def stop_countdown(self):
self.is_running = False
if self.timer_thread is not None:
self.timer_thread.join()
self.countdown.set(0)
self.update_text()
if __name__ == '__main__':
root = tk.Tk()
root.geometry('400x400')
timer = CountdownTimer(root)
root.mainloop()
```
在这个例子中,我们使用了tkinter创建了一个GUI界面,包括一个输入框、两个按钮和一个文本标签。当用户输入需要倒计时的时间后,点击开始按钮,就会创建一个子线程,使用while循环进行倒计时,并通过消息机制更新文本标签上的数字。当用户点击停止按钮时,程序会结束子线程并重置倒计时时间。
阅读全文
相关推荐
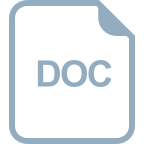
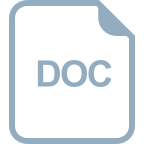
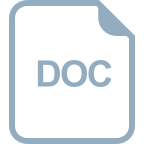
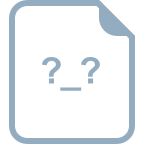
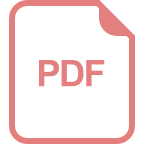
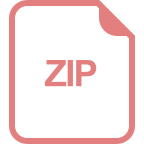
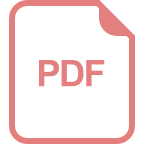
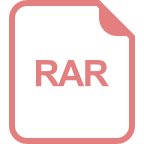
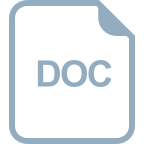
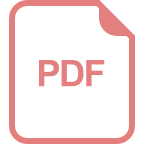








