Bin文件如何从CPU0发送到CPU1代码
时间: 2024-02-18 18:00:40 浏览: 27
以下是一个用C语言在Linux平台上实现将bin文件从CPU0发送到CPU1的示例代码:
CPU0发送代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/mman.h>
#define FILE_SIZE 4096
int main(int argc, char *argv[]) {
int fd;
char *src;
char *dst;
off_t offset = 0;
// 打开bin文件
if ((fd = open("test.bin", O_RDONLY)) < 0) {
perror("open");
exit(1);
}
// 映射bin文件到内存
src = mmap(NULL, FILE_SIZE, PROT_READ, MAP_PRIVATE, fd, offset);
if (src == MAP_FAILED) {
perror("mmap");
exit(1);
}
// 映射共享内存
int shm_fd = shm_open("/myshm", O_CREAT | O_RDWR, 0666);
if (shm_fd < 0) {
perror("shm_open");
exit(1);
}
if (ftruncate(shm_fd, FILE_SIZE) < 0) {
perror("ftruncate");
exit(1);
}
dst = mmap(NULL, FILE_SIZE, PROT_READ | PROT_WRITE, MAP_SHARED, shm_fd, 0);
if (dst == MAP_FAILED) {
perror("mmap");
exit(1);
}
// 复制bin文件内容到共享内存
memcpy(dst, src, FILE_SIZE);
// 解除内存映射
munmap(src, FILE_SIZE);
munmap(dst, FILE_SIZE);
// 关闭bin文件和共享内存
close(fd);
close(shm_fd);
return 0;
}
```
CPU1接收代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/mman.h>
#define FILE_SIZE 4096
int main(int argc, char *argv[]) {
char *src;
char *dst;
// 映射共享内存
int shm_fd = shm_open("/myshm", O_CREAT | O_RDWR, 0666);
if (shm_fd < 0) {
perror("shm_open");
exit(1);
}
dst = mmap(NULL, FILE_SIZE, PROT_READ | PROT_WRITE, MAP_SHARED, shm_fd, 0);
if (dst == MAP_FAILED) {
perror("mmap");
exit(1);
}
// 从共享内存中读取bin文件内容
src = malloc(FILE_SIZE);
memcpy(src, dst, FILE_SIZE);
// 解除内存映射
munmap(dst, FILE_SIZE);
// 写入bin文件
int fd;
if ((fd = open("test.bin", O_CREAT | O_WRONLY | O_TRUNC, 0666)) < 0) {
perror("open");
exit(1);
}
write(fd, src, FILE_SIZE);
// 释放内存和关闭文件
free(src);
close(fd);
close(shm_fd);
return 0;
}
```
这个示例代码中,CPU0打开bin文件,将其映射到内存中,并将其复制到共享内存中。CPU1打开共享内存,从中读取bin文件内容,并将其写入到新的bin文件中。需要注意的是,这里使用了Linux系统提供的共享内存机制,所以这个代码只能在Linux平台上运行,如果在其他平台上运行,需要使用其他的机制来实现进程间通信。
相关推荐
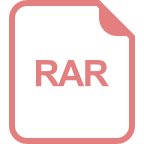
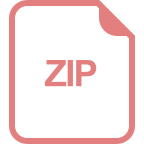
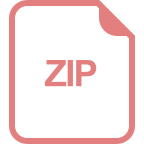









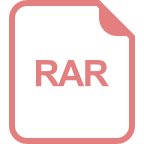
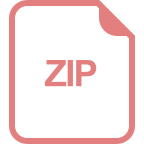
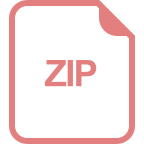
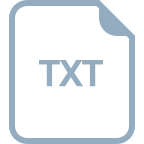
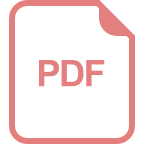
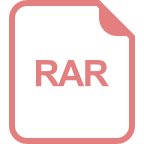