js编写校验身份证的程序
时间: 2024-06-11 19:08:29 浏览: 16
以下是一个简单的JavaScript程序,用于校验18位身份证号码的合法性:
```javascript
function validateIdCard(idCard) {
// 身份证号码位数不对或者格式不正确
if (idCard.length !== 18 || !/^\d{17}[\dXx]$/.test(idCard)) {
return false;
}
// 校验码校验
const chars = idCard.split('');
const factor = [7, 9, 10, 5, 8, 4, 2, 1, 6, 3, 7, 9, 10, 5, 8, 4, 2];
const parity = ['1', '0', 'X', '9', '8', '7', '6', '5', '4', '3', '2'];
let sum = 0;
for (let i = 0; i < 17; i++) {
sum += chars[i] * factor[i];
}
if (parity[sum % 11] !== chars[17].toUpperCase()) {
return false;
}
// 生日校验
const year = parseInt(idCard.substring(6, 10));
const month = parseInt(idCard.substring(10, 12));
const day = parseInt(idCard.substring(12, 14));
const date = new Date(year, month - 1, day);
if (
date.getFullYear() !== year ||
date.getMonth() !== month - 1 ||
date.getDate() !== day
) {
return false;
}
// 所有校验都通过,返回true
return true;
}
```
该函数接受一个身份证号码作为参数,返回一个布尔值,表示该身份证号码是否合法。
该函数的实现思路如下:
1. 首先判断身份证号码的长度和格式是否符合规范,如果不符合,则直接返回false;
2. 然后根据身份证号码的前17位和一个固定的因子进行校验码校验,如果校验码不正确,则返回false;
3. 接着根据身份证号码中的生日信息构造一个Date对象进行校验,如果生日不合法,则返回false;
4. 如果所有的校验都通过,则返回true。
注意,该函数只能校验18位身份证号码的合法性,不能校验15位身份证号码的合法性。如果需要校验15位身份证号码的合法性,需要对函数进行修改。
相关推荐
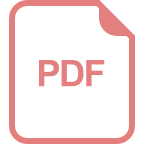
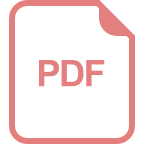














