添加 Jasypt 的加密盐值配置到 Tomcat
时间: 2023-11-22 07:04:59 浏览: 274
要在 Tomcat 中使用 Jasypt 的加密盐值配置,需要按以下步骤进行操作:
1. 将 Jasypt 的 jar 包添加到 Tomcat 的类路径中。
2. 在 Tomcat 的配置文件中添加以下代码:
```xml
<Context>
<Parameter name="jasypt.encryptor.password" value="your_password" override="false"/>
</Context>
```
其中,`your_password` 是你想要使用的加密盐值。
3. 在需要使用加密盐值的应用程序中,可以通过以下代码获取加密盐值:
```java
String password = System.getProperty("jasypt.encryptor.password");
```
注意,这种方式获取的加密盐值是加密的,需要使用 Jasypt 的 API 进行解密后才能使用。
以上就是在 Tomcat 中添加 Jasypt 的加密盐值配置的步骤。
相关问题
springboot jasypt加密解密配置
Spring Boot集成Jasypt用于安全地存储和处理敏感数据是一种常见的做法。以下是基本的配置步骤:
1. 添加依赖:首先,在你的`pom.xml`或`build.gradle`文件中添加Jasypt的Spring Boot starter依赖。
```xml
<!-- Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jasypt</artifactId>
</dependency>
// Gradle
implementation 'org.springframework.boot:spring-boot-starter-jasypt'
```
2. 配置属性:在`application.properties`或`application.yml`文件中设置相关的加密和解密参数。例如:
```properties
# 加密算法
spring.jasypt.encryptor.algorithm=BCrypt
# 密钥
spring.jasypt.encryptor.password=your_secret_key
# 如果使用环境变量替换硬编码的密钥
spring.jasypt.encryptor.key-alias=jasypt
# 明文源路径或资源名
spring.jasypt.encryptor.encrypt-context-headers=**/*.txt
```
3. 使用@Encryptable注解:如果你想对实体类字段进行加密,可以使用`@Encryptable`注解,并提供一个解密后的getter方法。
```java
import org.springframework.core.annotation.Encryptable;
@Entity
public class User {
@Encryptable
private String password;
//...
}
```
4. 解密服务:如果需要在Controller或Service中进行解密操作,你可以创建一个`PasswordEncoder`实现或使用Spring提供的默认实现:
```java
import org.springframework.context.annotation.Bean;
import org.springframework.stereotype.Component;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
@Component
public class CustomPasswordEncoder implements PasswordEncoder {
//... 实现PasswordEncoder接口的方法
}
```
5. 使用密码编码器:在处理用户注册或登录时,使用自定义的`PasswordEncoder`解码明文密码。
```java
@Autowired
private CustomPasswordEncoder passwordEncoder;
@PostMapping("/register")
public User register(@RequestBody User user) {
user.setPassword(passwordEncoder.encode(user.getPassword()));
return userService.save(user);
}
```
applicationContext.xml 中配置jasypt加密
`applicationContext.xml`是Spring框架中的一个核心配置文件,用于配置Spring的IoC容器,包括Bean的定义、依赖关系、数据源配置等。Jasypt(Java Simplified Encryption)是一个用于简化Java加密操作的库,它提供了一种方式,可以在不修改原有代码的情况下加密和解密敏感数据。
在`applicationContext.xml`中配置Jasypt加密,通常需要以下几个步骤:
1. 添加Jasypt依赖:首先需要在项目中添加Jasypt的依赖库。如果你使用Maven,可以在pom.xml文件中加入以下依赖:
```xml
<dependency>
<groupId>com.github.ulisesbocchio</groupId>
<artifactId>jasypt-spring-boot-starter</artifactId>
<version>你的版本号</version>
</dependency>
```
2. 配置加密属性:在`applicationContext.xml`中配置Jasypt所需的加密属性,例如加密算法名称、盐值(salt)等。
```xml
<bean id="jasyptConfig" class="org.jasypt.encryption.pbe.StandardPBEStringEncryptor">
<property name="algorithm" value="PBEWithHMACSHA512AndAES_256" />
<property name="password" value="你的密码" />
</bean>
```
3. 使用加密工具类:创建一个工具类,用于加密和解密属性值。
```java
public class JasyptUtil {
private static Encryptor encryptor = new StandardPBEStringEncryptor();
public static String encrypt(String valueToEncrypt) {
return encryptor.encrypt(valueToEncrypt);
}
public static String decrypt(String encryptedValue) {
return encryptor.decrypt(encryptedValue);
}
}
```
4. 在需要加密的属性上使用工具类:在配置文件中使用工具类对需要加密的属性值进行加密,然后将加密后的字符串放入`applicationContext.xml`。
```xml
<property name="yourProperty" value="${encrypted.yourProperty}" />
```
5. 使用`${}`语法引用加密后的值:在`applicationContext.xml`中使用`${}`语法引用加密后的属性值,Spring容器会自动调用Jasypt的加密工具类进行解密。
需要注意的是,随着版本的更新,Jasypt可能会提供更多简化配置的方式,建议参考最新的官方文档或GitHub上的说明进行配置。
阅读全文
相关推荐
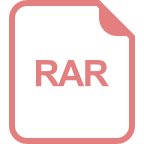
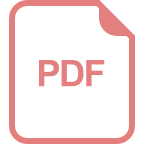
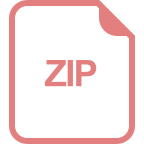
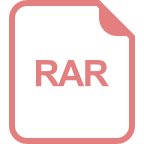
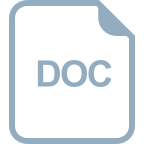
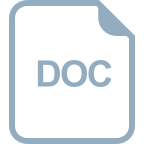







