请用rust帮我写一个提取应用程序图标的代码
时间: 2023-05-28 18:04:53 浏览: 454
以下是一个使用Rust提取应用程序图标的示例代码:
```rust
use std::fs::File;
use std::io::BufReader;
use image::GenericImageView;
fn extract_icon(file_path: &str) -> Option<image::DynamicImage> {
let file = match File::open(file_path) {
Ok(f) => f,
Err(_) => return None,
};
let reader = BufReader::new(file);
let zip = match zip::ZipArchive::new(reader) {
Ok(z) => z,
Err(_) => return None,
};
for i in 0..zip.len() {
let mut file = zip.by_index(i).unwrap();
let file_name = file.name().to_lowercase();
if file_name.ends_with(".ico") || file_name.ends_with(".exe") {
let mut buf = Vec::new();
if let Err(_) = file.read_to_end(&mut buf) {
continue;
}
match image::ico::IcoDecoder::new(&buf[..]) {
Ok(decoder) => {
if let Some(icon) = decoder.into_icons().next() {
return Some(icon);
}
},
Err(_) => continue,
}
match image::load_from_memory(&buf) {
Ok(image) => return Some(image),
Err(_) => continue,
}
}
}
None
}
fn main() {
let icon = extract_icon("path/to/file.exe").unwrap();
println!("Icon width: {}, height: {}", icon.width(), icon.height());
}
```
该代码打开一个应用程序文件(.ico或.exe),解析其内容以查找图标。如果找到,它将返回`image::DynamicImage`类型的图像对象。如果找不到或解析失败,则返回`None`。请注意,此代码依赖于`image`和`zip` crate。
阅读全文
相关推荐
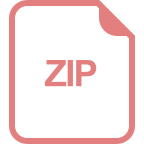
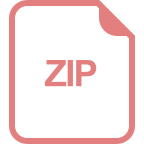
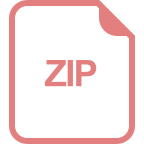
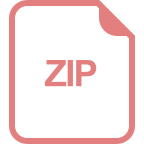
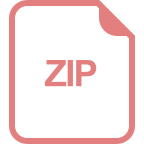
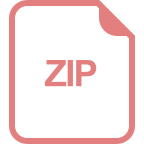
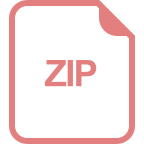
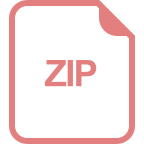
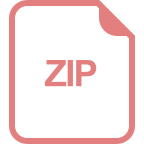
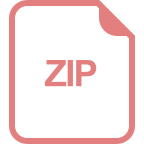
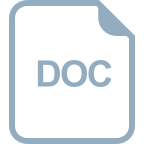
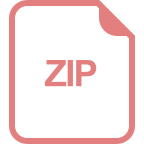
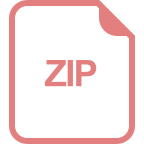
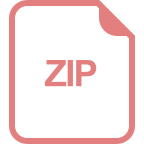
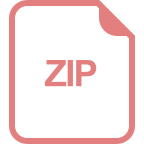