ffmpeg怎么播放QByteArray数据
时间: 2023-07-08 11:29:07 浏览: 98
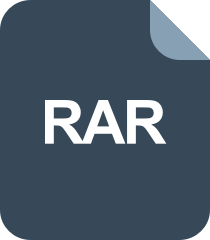
FFMPEG播放RTSP视频流
为了在ffmpeg中播放QByteArray数据,您需要将其转换为AVPacket结构体,然后将其传递给ffmpeg进行解码和播放。以下是一个简单的示例代码:
```C++
#include <QByteArray>
#include <QDebug>
extern "C" {
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
}
void playQByteArrayData(const QByteArray &data)
{
// 初始化ffmpeg
av_register_all();
// 创建解码器上下文
AVCodec *codec = avcodec_find_decoder(AV_CODEC_ID_MP3);
AVCodecContext *codecCtx = avcodec_alloc_context3(codec);
avcodec_open2(codecCtx, codec, NULL);
// 创建格式化上下文
AVFormatContext *formatCtx = avformat_alloc_context();
AVIOContext *ioCtx = avio_alloc_context(
(unsigned char *)data.data(), data.size(), 0, NULL, NULL, NULL, NULL);
formatCtx->pb = ioCtx;
// 查找音频流信息
avformat_find_stream_info(formatCtx, NULL);
int audioStreamIndex = -1;
for (int i = 0; i < formatCtx->nb_streams; i++) {
if (formatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_AUDIO) {
audioStreamIndex = i;
break;
}
}
if (audioStreamIndex == -1) {
qDebug() << "Failed to find audio stream.";
return;
}
// 创建解码器
AVCodecParameters *codecParams = formatCtx->streams[audioStreamIndex]->codecpar;
AVCodec *decoder = avcodec_find_decoder(codecParams->codec_id);
AVCodecContext *decoderCtx = avcodec_alloc_context3(decoder);
avcodec_parameters_to_context(decoderCtx, codecParams);
avcodec_open2(decoderCtx, decoder, NULL);
// 创建重采样上下文
SwrContext *swrCtx = swr_alloc();
av_opt_set_int(swrCtx, "in_channel_layout", decoderCtx->channel_layout, 0);
av_opt_set_int(swrCtx, "out_channel_layout", AV_CH_LAYOUT_STEREO, 0);
av_opt_set_int(swrCtx, "in_sample_rate", decoderCtx->sample_rate, 0);
av_opt_set_int(swrCtx, "out_sample_rate", decoderCtx->sample_rate, 0);
av_opt_set_sample_fmt(swrCtx, "in_sample_fmt", decoderCtx->sample_fmt, 0);
av_opt_set_sample_fmt(swrCtx, "out_sample_fmt", AV_SAMPLE_FMT_FLTP, 0);
swr_init(swrCtx);
// 解码并重采样音频数据
AVPacket packet;
av_init_packet(&packet);
AVFrame *frame = av_frame_alloc();
while (av_read_frame(formatCtx, &packet) >= 0) {
if (packet.stream_index == audioStreamIndex) {
avcodec_send_packet(decoderCtx, &packet);
while (avcodec_receive_frame(decoderCtx, frame) == 0) {
AVFrame *resampledFrame = av_frame_alloc();
resampledFrame->nb_samples = frame->nb_samples;
resampledFrame->format = AV_SAMPLE_FMT_FLTP;
resampledFrame->channel_layout = AV_CH_LAYOUT_STEREO;
av_frame_get_buffer(resampledFrame, 0);
swr_convert(swrCtx, resampledFrame->data, resampledFrame->nb_samples,
(const uint8_t **)frame->data, frame->nb_samples);
// 在此处可以将音频数据传递给音频播放器进行播放
av_frame_free(&resampledFrame);
}
}
av_packet_unref(&packet);
}
// 释放资源
av_frame_free(&frame);
avcodec_free_context(&decoderCtx);
avformat_close_input(&formatCtx);
avio_context_free(&ioCtx);
avcodec_free_context(&codecCtx);
}
```
此代码将QByteArray数据作为输入,并使用ffmpeg进行解码和重采样,然后可以将音频数据传递给音频播放器进行播放。请注意,此示例仅演示了如何使用ffmpeg解码和重采样音频数据,您需要根据自己的需求将音频数据传递给适当的音频播放器进行播放。
阅读全文
相关推荐
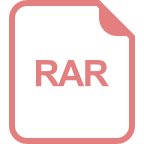
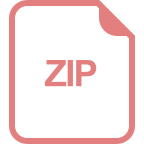















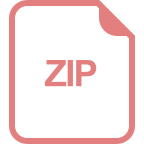