cpp中使用数值微分(dda)算法实现直线扫描转换需要代码示例
时间: 2024-10-18 14:19:42 浏览: 48
在C++中,数值微分(DDA,Double Differencing Algorithm)是一种简单但低精度的方式来近似函数的一阶导数,用于计算图像像素斜率。它主要用于教学目的和简单的图形处理应用。下面是一个基本的DDA算法实现直线扫描转换的例子:
```cpp
#include <iostream>
#include <cmath>
// 定义线段起点和终点
struct Point {
int x, y;
};
// 计算两点之间的斜率
double dda_slope(const Point& start, const Point& end) {
if (start.x == end.x)
return std::numeric_limits<double>::infinity(); // 平行x轴,斜率为无穷大
else
return (double)(end.y - start.y) / (end.x - start.x);
}
// 使用DDA算法进行扫描转换
void scanconvert(const Point& start, const Point& end) {
double dx = end.x - start.x;
double dy = end.y - start.y;
for (int x = start.x; x <= end.x; ++x) {
for (int y = start.y; y <= end.y; ++y) { // 这里假设我们要遍历整个线段内的像素点
double slope = dda_slope({x, start.y}, {x, y}); // 根据当前x坐标计算斜率
if (slope != std::numeric_limits<double>::infinity()) { // 如果不是平行x轴的线
// 按照斜率计算增量,调整y值
int pixel_y = start.y + static_cast<int>(slope * (x - start.x));
// 然后绘制到屏幕或其他目的地
draw_pixel(x, pixel_y); // 假设draw_pixel是一个实际函数,用于绘制像素
}
}
}
}
阅读全文
相关推荐
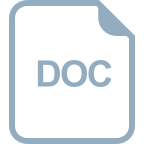
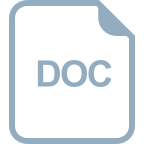
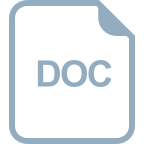

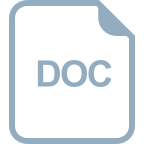
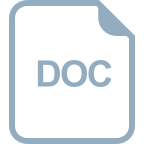
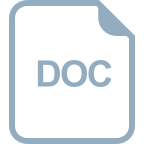
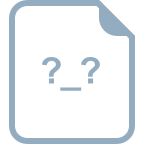
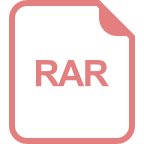
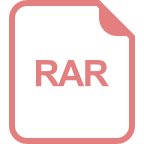
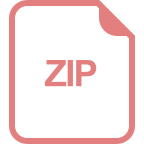
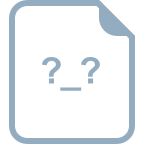
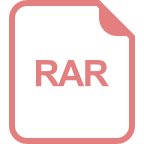
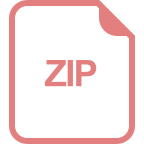
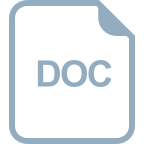
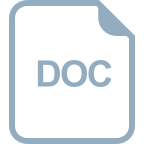
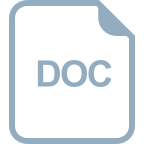
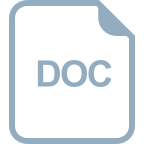
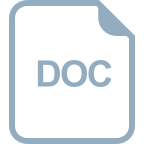