用C语言从键盘读入字符(数字、字母或其他符号)建立一个深度不小于3层的树的孩子兄弟表存储结构,然后①分别递归先根、后根遍历该树并输出相应遍历树结点序列。
时间: 2023-06-19 19:03:05 浏览: 56
下面是基于孩子兄弟表存储结构的 C 语言代码实现,包括建立树和先根、后根遍历输出:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct TreeNode {
char data;
struct TreeNode *firstChild;
struct TreeNode *nextSibling;
} TreeNode, *TreeNodePtr;
TreeNodePtr createNode(char data) {
TreeNodePtr node = (TreeNodePtr)malloc(sizeof(TreeNode));
node->data = data;
node->firstChild = NULL;
node->nextSibling = NULL;
return node;
}
TreeNodePtr createTree() {
char data;
scanf("%c", &data);
getchar(); //读入回车符
if (data == '\n') {
return NULL;
}
TreeNodePtr root = createNode(data);
TreeNodePtr parent = root;
int depth = 0;
while (depth >= 0) {
scanf("%c", &data);
getchar(); //读入回车符
if (data == '\n') {
depth--;
if (parent != NULL) {
parent = parent->nextSibling;
}
} else if (data == '#') {
depth++;
if (parent != NULL) {
parent = parent->firstChild;
}
} else {
TreeNodePtr node = createNode(data);
if (parent->firstChild == NULL) {
parent->firstChild = node;
} else {
TreeNodePtr sibling = parent->firstChild;
while (sibling->nextSibling != NULL) {
sibling = sibling->nextSibling;
}
sibling->nextSibling = node;
}
}
}
return root;
}
void preOrder(TreeNodePtr root) {
if (root != NULL) {
printf("%c ", root->data);
preOrder(root->firstChild);
preOrder(root->nextSibling);
}
}
void postOrder(TreeNodePtr root) {
if (root != NULL) {
postOrder(root->firstChild);
printf("%c ", root->data);
postOrder(root->nextSibling);
}
}
int main() {
TreeNodePtr root = createTree();
printf("先根遍历结果:");
preOrder(root);
printf("\n后根遍历结果:");
postOrder(root);
printf("\n");
return 0;
}
```
使用时,输入字符序列时,每个节点的数据用一个字符表示,节点之间用空格分隔,每一层结束时用一个 # 符号表示,每个节点的子节点用多个回车符表示。例如,下面是一个输入样例:
```
A
B #
C D #
E F #
G # #
```
表示建立了一个如下的树:
```
A
/ \
B G
/ \
C D
/ \
E F
```
输出结果为:
```
先根遍历结果:A B C D E F G
后根遍历结果:C E F D B G A
```
相关推荐
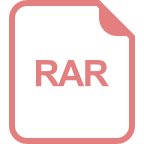
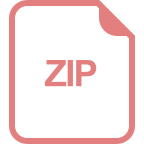














