c++ qt5.9 mysql 使用QDataWidgetMapper 查询 提交 数据
时间: 2024-05-15 19:13:28 浏览: 127
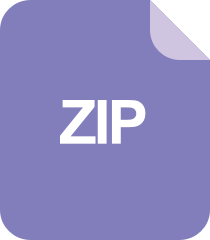
QDataWidgetMapper
QDataWidgetMapper 是一个非常方便的工具,可以用来将数据库中的数据映射到Qt中的控件上,也可以将Qt中的控件上的数据提交到数据库中。
下面是一个简单的示例,演示如何使用QDataWidgetMapper来查询和提交数据。
首先,需要在Qt中连接到MySQL数据库。可以使用Qt提供的QSqlDatabase类来实现。以下是一个简单的连接示例:
```c++
QSqlDatabase db = QSqlDatabase::addDatabase("QMYSQL");
db.setHostName("localhost");
db.setDatabaseName("mydatabase");
db.setUserName("myusername");
db.setPassword("mypassword");
if (!db.open()) {
qDebug() << "Failed to connect to database";
}
```
接下来,需要创建一个QSqlTableModel对象,用于从数据库中检索数据:
```c++
QSqlTableModel *model = new QSqlTableModel(this, db);
model->setTable("mytable");
model->select();
```
现在,可以将QSqlTableModel对象中的数据映射到Qt中的控件上。为此,需要创建一个QDataWidgetMapper对象,并将其与控件和模型对象关联:
```c++
QDataWidgetMapper *mapper = new QDataWidgetMapper(this);
mapper->setModel(model);
mapper->addMapping(ui->lineEditName, 1); // Map column 1 to line edit
mapper->addMapping(ui->lineEditAge, 2); // Map column 2 to line edit
mapper->toFirst(); // Map data from the first row
```
现在,可以使用QDataWidgetMapper对象查询和显示数据。以下是一个简单的查询示例:
```c++
mapper->toFirst(); // Move to the first row
while (mapper->currentIndex() != -1) {
QString name = mapper->mappedWidgetAt(0)->property("text").toString();
int age = mapper->mappedWidgetAt(1)->property("text").toInt();
qDebug() << name << age;
mapper->toNext(); // Move to the next row
}
```
要提交数据,只需在QDataWidgetMapper对象上调用submit()方法即可:
```c++
mapper->submit();
```
如果需要撤消所有未提交的更改,可以使用revert()方法:
```c++
mapper->revert();
```
希望这个示例对你有所帮助!
阅读全文
相关推荐





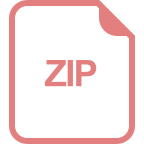
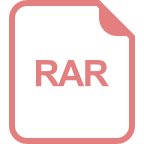
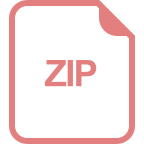
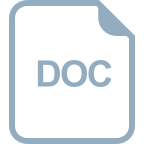
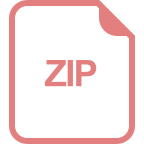
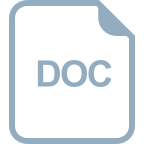


