/workspace/CppProject/main.cpp:1:10: error: expected "FILENAME" or <FILENAME> #import random rules = (1: 3, 2: 1, 3: 2)
时间: 2024-02-02 19:05:05 浏览: 165
这是因为你使用了错误的导入语句。在 C++ 中,应该使用 `#include` 来导入头文件,而不是 `#import`。另外,在 C++ 中,使用大括号表示字典是不被允许的,你可以使用 `std::map` 来代替。下面是一个示例代码:
```cpp
#include <iostream>
#include <map>
#include <cstdlib>
#include <ctime>
using namespace std;
int main() {
// 定义游戏规则
map<int, int> rules = {{1, 3}, {2, 1}, {3, 2}};
// 记录用户和计算机的得分
int user_score = 0;
int computer_score = 0;
int draws = 0;
// 进行游戏
srand(time(NULL));
for (int i = 0; i < 3; i++) {
// 计算机随机生成一个数(1、2、3分别代表剪刀、石头、布)
int computer_choice = rand() % 3 + 1;
// 用户输入自己的选择(1、2、3分别代表剪刀、石头、布)
int user_choice;
cout << "请出拳(1代表剪刀,2代表石头,3代表布):";
cin >> user_choice;
// 判断胜负
int result = rules[user_choice] - computer_choice;
if (result == 0) {
cout << "第" << i+1 << "局:势均力敌" << endl;
draws++;
} else if (result == 1 || result == -2) {
cout << "第" << i+1 << "局:您赢了" << endl;
user_score++;
} else {
cout << "第" << i+1 << "局:您输了" << endl;
computer_score++;
}
}
// 输出最终结果
cout << "游戏结束,您赢了" << user_score << "局,输了" << computer_score << "局,平了" << draws << "局" << endl;
return 0;
}
```
在这个示例代码中,使用了 `std::map` 来代替字典,使用了 `std::srand()` 和 `std::rand()` 来生成随机数,使用了 `std::cin` 和 `std::cout` 来进行输入输出。
阅读全文
相关推荐





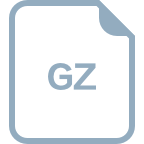
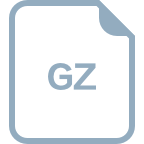








