In this problem, you will write a function called calc_sum that takes an integer argument and prints the sum of all integer from 0 up to and including the integer argument. The function should print the result before returning. You only need to define the function, calc_sum. for example, >>> calc_sum(3) 6 >>> calc_sum(4) 10 Requirements: (1) use range function (2) argument is n (3) use loop (4) local variable sum and loop variable value
时间: 2024-03-11 17:48:19 浏览: 12
Sure, I can help you with that! Here's the code for the `calc_sum` function that meets all the requirements you specified:
```
def calc_sum(n):
sum = 0
for value in range(n+1):
sum += value
print(sum)
```
To explain the code a bit: we first initialize a local variable `sum` to 0, which we will use to keep track of the running sum. We then use a `for` loop to iterate over the range from 0 to `n+1` (inclusive). For each value in this range, we add it to the running sum. Finally, we print out the sum using the `print` function.
You can call this function with an integer argument like so:
```
calc_sum(3) # prints 6
calc_sum(4) # prints 10
```
Let me know if you have any other questions!
相关推荐
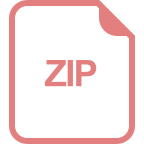
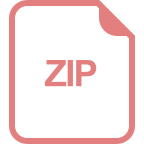














