c#编写计算n!,能捕捉算术溢出异常,并能输出产生溢出时的n值。
时间: 2024-03-09 07:49:00 浏览: 31
好的,下面是一个示例的C#代码实现:
```
public static int Factorial(int n) {
int result = 1;
for (int i = 1; i <= n; i++) {
try {
result = checked(result * i);
} catch (System.OverflowException e) {
Console.WriteLine("产生算术溢出的n值为:" + i);
return -1;
}
}
return result;
}
```
该方法使用了`checked`关键字来捕捉算术溢出异常,如果在计算时出现了溢出,就会抛出`System.OverflowException`异常。在循环中,如果捕获到了该异常,就会输出产生溢出的n值,并且返回-1表示计算失败。如果没有出现溢出,则返回计算结果。
同样需要注意的是,该实现只能处理n的值小于等于12的情况,因为当n大于12时,计算结果会超出int类型的范围,无法正确计算。如果需要计算更大的阶乘,需要使用更大的数据类型或者其他算法。
相关问题
用c#编写计算n!,能捕捉算术溢出异常,并能输出产生溢出时的n值。(设n为int型)
可以使用`BigInteger`类型来避免算术溢出,同时使用`try-catch`语句捕捉溢出异常,代码如下:
```csharp
using System;
using System.Numerics;
class Program
{
static BigInteger Factorial(int n)
{
BigInteger result = 1;
for (int i = 1; i <= n; i++)
{
try
{
result *= i;
if (result < 0)
{
throw new OverflowException("Factorial overflow");
}
}
catch (OverflowException ex)
{
Console.WriteLine($"{ex.Message}: n = {i}");
return -1;
}
}
return result;
}
static void Main(string[] args)
{
int n = 25;
BigInteger result = Factorial(n);
if (result != -1)
{
Console.WriteLine($"{n}! = {result}");
}
}
}
```
在上面的代码中,我们使用`BigInteger`类型存储阶乘的结果,然后在每次乘法操作后判断结果是否小于0,如果小于0则说明发生了算术溢出,此时我们使用`throw`语句抛出一个`OverflowException`异常,然后在`catch`语句中捕获该异常并输出提示信息。最后的结果如果不为-1,则说明计算成功,输出阶乘的值。
使用C#编写一个控制台应用程序,从控制台输入要查找的关键字,并在指定文档中查找该关键字,打印输出关键字所在的那一行语句,最后统计该关键字在全文中出现的次数
编程语言的优缺点是什么?
C语言是一种高效、灵活、跨平台的编程语言,具有以下优点:
1. 产生高效的代码,运行速度快。
2. 可以方便地访问操作系统内核的功能。
3. 语法简单,容易学习和理解。
4. 对内存的管理灵活,可以手动管理内存,实现精细化的资源控制。
5. 在编译期间就能够发现一些编程错误,提高了程序的稳定性和可靠性。
然而,C语言也存在一些缺点:
1. 容易出现内存泄漏和指针相关的运行错误。
2. 缺乏现代面向对象和函数式编程等一些高级编程特性。
3. 开发周期较长,需要手动管理内存,在开发复杂大型系统时可能会花费较长时间。
4. 容易受到缓冲区溢出、算术异常等攻击,对安全性的保护需要额外的措施。
总的来说,C语言是一种底层语言,虽然功能强大、效率高,但需要程序员具备一些底层编程知识和经验。
相关推荐
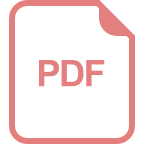
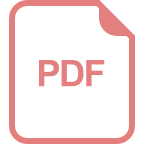
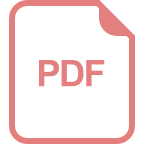







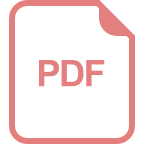
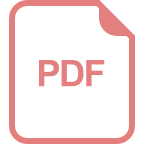
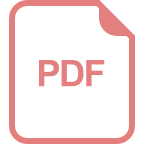
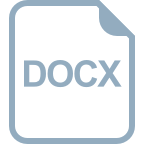
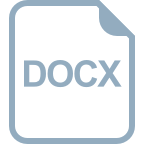
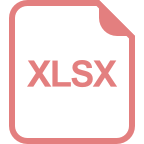