openmv 用串口发送jgeg格式图像
时间: 2023-11-30 17:05:24 浏览: 33
OpenMV支持使用串口发送JPEG格式的图像,可以使用`sensor.snapshot()`函数获取图像帧,然后使用`image.compress()`函数将图像压缩为JPEG格式。最后,使用串口发送函数将压缩后的JPEG数据发送出去即可。
以下是一个示例代码,通过串口将压缩后的JPEG数据发送出去:
```python
import sensor, image, time, ustruct
from pyb import UART
# 初始化串口
uart = UART(3, 115200)
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time=2000)
while(True):
# 获取摄像头图像帧
img = sensor.snapshot()
# 压缩图像为JPEG
buf = ustruct.pack("<i", img.size())
buf += image.compress(img)
# 发送JPEG数据
uart.write(buf)
```
在上述代码中,我们将压缩后的JPEG数据打包为一个字节数组`buf`,其中前四个字节为图像数据长度,后面的字节为压缩后的JPEG数据。最后,我们使用串口的`write()`函数将数据发送出去。
在接收端,你需要解析接收到的数据,并将其转换为JPEG图像。具体实现方式可以参考以下代码:
```python
import ustruct, sensor, image
from pyb import UART
# 初始化串口
uart = UART(3, 115200)
while(True):
# 读取JPEG数据长度
len_bytes = uart.read(4)
if len_bytes:
# 解析JPEG数据长度并读取JPEG数据
img_len = ustruct.unpack("<i", len_bytes)[0]
img_bytes = uart.read(img_len)
# 将JPEG数据转换为图像
img = image.ImageIO(img_bytes)
# 显示图像
sensor.flush()
img.display()
```
在上述代码中,我们首先读取四个字节的数据作为JPEG数据长度,然后读取对应长度的数据作为JPEG数据。接着,我们使用`ImageIO`类将JPEG数据转换为图像,并使用`display()`函数显示图像。
相关推荐
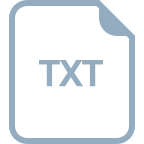
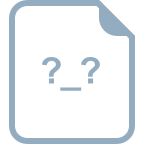
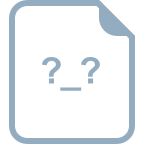
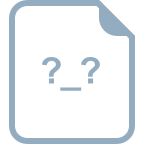
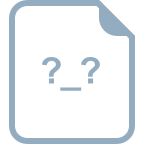
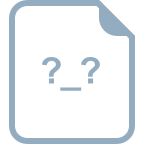
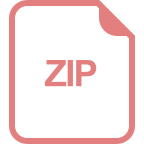
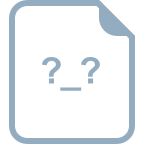
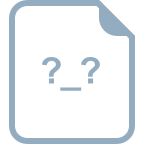
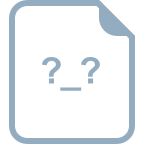
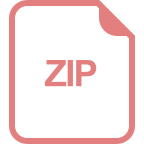