以下是使用 from_utf8_lossy 方法的示例代码:
时间: 2024-11-23 13:40:45 浏览: 5
`from_utf8_lossy` 是一个处理字符串编码转换的方法,通常用于Python的`chardet`库中,该库用于检测文本的字符编码。这个方法会尝试将字节序列解码成UTF-8格式,如果遇到无法识别的字符,它会选择丢弃而不是引发错误。这在处理可能存在未知字符的二进制数据时很有用,因为不是所有字符都能准确地映射到UTF-8。
下面是一个简单的示例:
```python
import chardet
# 假设我们有一个可能是UTF-8编码的字节串
byte_string = b'\xd0\xbf\xd1\x80\xd0\xb5\xd0\xb4\xd0\xb5\xd0\xbd\xd0\xb8\xd1\x8f'
# 使用 from_utf8_lossy 进行解码
detected_encoding = chardet.detect(byte_string)
try:
decoded_text = byte_string.decode(detected_encoding['encoding'], 'from_utf8_lossy')
except UnicodeDecodeError:
print("Failed to decode with lossy method")
print(decoded_text) # 输出解码后的文本,可能包含不可识别的字符
```
相关问题
java: incompatible types: possible lossy conversion from long to int
This error occurs when you try to assign a long value to an int variable, and the long value is too large to be represented as an int. In Java, long is a 64-bit signed integer, while int is a 32-bit signed integer. Therefore, if you try to assign a long value that is larger than the maximum value of an int (2147483647), the compiler will generate an error.
For example, the following code will generate the error "incompatible types: possible lossy conversion from long to int":
```
long myLong = 1234567890;
int myInt = myLong;
```
To fix this error, you can either change the type of the variable to long, or you can cast the long value to an int using the (int) operator. However, be aware that casting a long value to an int may result in data loss, as the long value may be too large to fit into the int variable.
error: incompatible types: possible lossy conversion from long to int
这个错误通常是因为试图将一个 long 类型的值赋给一个 int 类型的变量,而 int 类型的范围比 long 类型小,因此可能会发生数据丢失。要解决这个问题,可以将 int 类型的变量改为 long 类型,或者使用强制类型转换将 long 类型的值转换为 int 类型。例如:
```
long num1 = 10000000000L;
int num2 = (int) num1;
```
在这个例子中,num1 是 long 类型的,num2 是 int 类型的,我们使用强制类型转换将 num1 转换为 int 类型的值并赋给 num2。请注意,由于 num1 的值超过了 int 类型的范围,因此我们在其后面添加了一个大写字母 L,表示这是一个 long 类型的字面量。
阅读全文
相关推荐
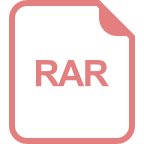
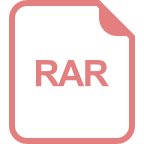
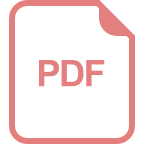













