android写一个发送广播和接收广播的完整代码
时间: 2023-07-29 11:08:05 浏览: 95
下面是一个简单的发送和接收自定义广播的完整代码示例:
发送广播:
```java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 发送自定义广播
Intent intent = new Intent("com.example.MY_CUSTOM_ACTION");
intent.putExtra("message", "Hello world!");
sendBroadcast(intent);
}
}
```
接收广播:
```java
public class MyBroadcastReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
if (intent.getAction().equals("com.example.MY_CUSTOM_ACTION")) {
// 获取广播中的消息
String message = intent.getStringExtra("message");
Toast.makeText(context, message, Toast.LENGTH_SHORT).show();
}
}
}
```
在 AndroidManifest.xml 文件中注册广播接收器:
```xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.broadcastdemo">
<uses-permission android:name="android.permission.INTERNET"/>
<application
...>
<receiver android:name=".MyBroadcastReceiver">
<intent-filter>
<action android:name="com.example.MY_CUSTOM_ACTION"/>
</intent-filter>
</receiver>
</application>
</manifest>
```
注意:在 AndroidManifest.xml 文件中注册的广播接收器只能接收应用程序在前台或后台运行时发送的广播。如果应用程序被销毁,则无法接收广播。如果需要在应用程序未运行时接收广播,则需要使用动态注册方式注册广播接收器。
阅读全文
相关推荐
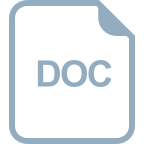
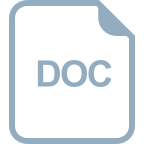
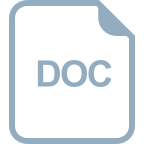
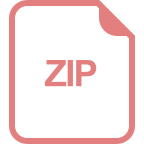
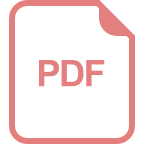
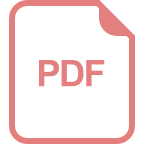
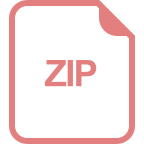
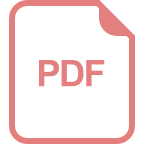
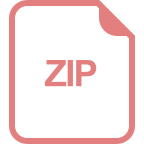
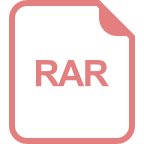
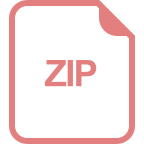
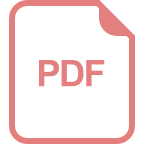
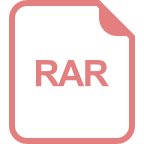
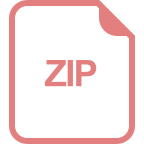
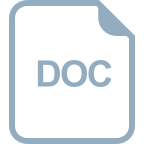
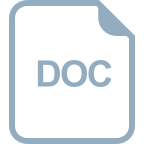
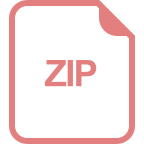