Android app 怎么拿到usb中的视频文件
时间: 2024-03-13 09:47:08 浏览: 22
要在 Android 应用程序中读取 USB 设备中的视频文件,可以基于上面提到的步骤,进行以下几个步骤:
1. 确定要读取的视频文件路径。
可以使用 UsbFile 类来访问 USB 设备中的文件系统,示例代码如下:
```
UsbFile root = UsbFileStreamFactory.create(usbFilesystem.getVolume(0).getRoot(), usbFilesystem);
UsbFile videoFile = root.search("video.mp4");
```
2. 使用 MediaPlayer 播放视频文件。
可以使用 MediaPlayer 类来播放视频文件,示例代码如下:
```
MediaPlayer mediaPlayer = new MediaPlayer();
mediaPlayer.setDataSource(new UsbFileInputStream(videoFile).getFD());
mediaPlayer.prepare();
mediaPlayer.start();
```
请注意,以上代码仅为示例代码,实际开发中需要根据具体情况进行调整。另外,需要使用第三方库来访问 USB 文件系统,比如 usb-serial-for-android 或者 libaums。同时,还需要在 AndroidManifest.xml 文件中添加适当的权限,比如访问存储权限。
相关问题
Android app 怎么拿到usb中的文件
要在 Android 应用程序中读取 USB 设备中的文件,需要进行以下几个步骤:
1. 在 AndroidManifest.xml 文件中添加 USB 权限:
```
<uses-feature android:name="android.hardware.usb.host" />
<uses-permission android:name="android.permission.USB_PERMISSION" />
```
2. 检查是否连接了 USB 设备:
可以使用 UsbManager 类来获取当前连接的 USB 设备列表,示例代码如下:
```
UsbManager usbManager = (UsbManager) getSystemService(Context.USB_SERVICE);
HashMap<String, UsbDevice> deviceList = usbManager.getDeviceList();
```
3. 请求 USB 权限:
当应用程序需要访问 USB 设备时,需要请求 USB 权限。示例代码如下:
```
PendingIntent permissionIntent = PendingIntent.getBroadcast(this, 0, new Intent(ACTION_USB_PERMISSION), 0);
usbManager.requestPermission(device, permissionIntent);
```
4. 读取文件:
可以使用 UsbFile 类来读取 USB 设备中的文件,示例代码如下:
```
UsbFileInputStream inputStream = new UsbFileInputStream(file);
byte[] buffer = new byte[1024];
int len;
while ((len = inputStream.read(buffer)) > 0) {
// 处理读取到的数据
}
```
请注意,以上代码仅为示例代码,实际开发中需要根据具体情况进行调整。另外,需要使用第三方库来访问 USB 文件系统,比如 usb-serial-for-android 或者 libaums。
android app弹出访问usb设备
要在Android应用程序中访问USB设备,您需要执行以下步骤:
1. 在AndroidManifest.xml文件中添加以下权限:
```
<uses-feature android:name="android.hardware.usb.host" />
<uses-permission android:name="android.permission.USB_PERMISSION" />
```
2. 检测连接的USB设备:
```
UsbManager usbManager = (UsbManager) getSystemService(Context.USB_SERVICE);
HashMap<String, UsbDevice> usbDevices = usbManager.getDeviceList();
if (!usbDevices.isEmpty()) {
// USB设备已连接
UsbDevice device = usbDevices.get(deviceName);
PendingIntent pendingIntent = PendingIntent.getBroadcast(this, 0, new Intent(ACTION_USB_PERMISSION), 0);
usbManager.requestPermission(device, pendingIntent);
} else {
// 没有连接的USB设备
}
```
3. 处理用户授权:
```
private static final String ACTION_USB_PERMISSION = "com.android.example.USB_PERMISSION";
BroadcastReceiver usbReceiver = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (ACTION_USB_PERMISSION.equals(action)) {
synchronized (this) {
UsbDevice device = (UsbDevice) intent.getParcelableExtra(UsbManager.EXTRA_DEVICE);
if (intent.getBooleanExtra(UsbManager.EXTRA_PERMISSION_GRANTED, false)) {
if (device != null) {
// 授权成功,可以开始访问USB设备
}
} else {
// 用户拒绝了授权请求
}
}
}
}
};
registerReceiver(usbReceiver, new IntentFilter(ACTION_USB_PERMISSION));
```
4. 访问USB设备:
```
UsbDeviceConnection connection = usbManager.openDevice(device);
UsbInterface usbInterface = device.getInterface(0);
UsbEndpoint endpoint = usbInterface.getEndpoint(0);
connection.claimInterface(usbInterface, true);
byte[] buffer = new byte[endpoint.getMaxPacketSize()];
int count = connection.bulkTransfer(endpoint, buffer, buffer.length, TIMEOUT);
```
请注意,这仅是一个简单的示例,并且需要根据您的具体需求进行修改和优化。
相关推荐
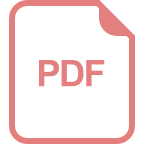












