写一个完善的android计算器代码
时间: 2024-03-12 10:44:30 浏览: 16
下面是一个完善的Android计算器代码,实现简单的加、减、乘、除功能,以及清除和删除功能:
```java
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private TextView tvResult;
private String currentNumber = "";
private String lastOperation = "";
private double result = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tvResult = findViewById(R.id.tvResult);
findViewById(R.id.btn0).setOnClickListener(this);
findViewById(R.id.btn1).setOnClickListener(this);
findViewById(R.id.btn2).setOnClickListener(this);
findViewById(R.id.btn3).setOnClickListener(this);
findViewById(R.id.btn4).setOnClickListener(this);
findViewById(R.id.btn5).setOnClickListener(this);
findViewById(R.id.btn6).setOnClickListener(this);
findViewById(R.id.btn7).setOnClickListener(this);
findViewById(R.id.btn8).setOnClickListener(this);
findViewById(R.id.btn9).setOnClickListener(this);
findViewById(R.id.btnAdd).setOnClickListener(this);
findViewById(R.id.btnSubtract).setOnClickListener(this);
findViewById(R.id.btnMultiply).setOnClickListener(this);
findViewById(R.id.btnDivide).setOnClickListener(this);
findViewById(R.id.btnClear).setOnClickListener(this);
findViewById(R.id.btnDelete).setOnClickListener(this);
findViewById(R.id.btnEqual).setOnClickListener(this);
}
@Override
public void onClick(View view) {
switch (view.getId()) {
case R.id.btn0:
currentNumber += "0";
tvResult.setText(currentNumber);
break;
case R.id.btn1:
currentNumber += "1";
tvResult.setText(currentNumber);
break;
case R.id.btn2:
currentNumber += "2";
tvResult.setText(currentNumber);
break;
case R.id.btn3:
currentNumber += "3";
tvResult.setText(currentNumber);
break;
case R.id.btn4:
currentNumber += "4";
tvResult.setText(currentNumber);
break;
case R.id.btn5:
currentNumber += "5";
tvResult.setText(currentNumber);
break;
case R.id.btn6:
currentNumber += "6";
tvResult.setText(currentNumber);
break;
case R.id.btn7:
currentNumber += "7";
tvResult.setText(currentNumber);
break;
case R.id.btn8:
currentNumber += "8";
tvResult.setText(currentNumber);
break;
case R.id.btn9:
currentNumber += "9";
tvResult.setText(currentNumber);
break;
case R.id.btnAdd:
performOperation();
lastOperation = "+";
break;
case R.id.btnSubtract:
performOperation();
lastOperation = "-";
break;
case R.id.btnMultiply:
performOperation();
lastOperation = "*";
break;
case R.id.btnDivide:
performOperation();
lastOperation = "/";
break;
case R.id.btnClear:
clear();
break;
case R.id.btnDelete:
delete();
break;
case R.id.btnEqual:
calculate();
break;
}
}
private void performOperation() {
if (!currentNumber.isEmpty()) {
double number = Double.parseDouble(currentNumber);
if (result == 0) {
result = number;
} else {
switch (lastOperation) {
case "+":
result += number;
break;
case "-":
result -= number;
break;
case "*":
result *= number;
break;
case "/":
result /= number;
break;
}
}
currentNumber = "";
}
}
private void calculate() {
if (!currentNumber.isEmpty()) {
performOperation();
}
tvResult.setText(String.valueOf(result));
result = 0;
lastOperation = "";
}
private void clear() {
currentNumber = "";
result = 0;
lastOperation = "";
tvResult.setText("");
}
private void delete() {
if (!currentNumber.isEmpty()) {
currentNumber = currentNumber.substring(0, currentNumber.length() - 1);
tvResult.setText(currentNumber);
}
}
}
```
在xml中,我们可以使用如下代码布局:
```xml
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/tvResult"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="24sp"
android:padding="16dp"
android:background="@color/colorPrimaryDark"
android:textColor="@android:color/white"
android:gravity="right"/>
<GridLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:rowCount="5"
android:columnCount="4"
android:padding="8dp">
<Button
android:id="@+id/btnClear"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="C"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btnDelete"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="DEL"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btnDivide"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="/"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btnMultiply"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="×"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btn7"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="7"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btn8"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="8"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btn9"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="9"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btnSubtract"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="-"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btn4"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="4"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btn5"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="5"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btn6"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="6"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btnAdd"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="+"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btn1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="1"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btn2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="2"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btn3"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="3"
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btnEqual"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="="
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="2"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btn0"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="0"
android:textSize="24sp"
android:layout_columnWeight="2"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
<Button
android:id="@+id/btnDecimal"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="."
android:textSize="24sp"
android:layout_columnWeight="1"
android:layout_rowWeight="1"
android:layout_gravity="fill"/>
</GridLayout>
</LinearLayout>
```
这里使用了GridLayout来实现按钮的布局,代码中使用了字符串转换成数字的方法实现计算功能。
相关推荐
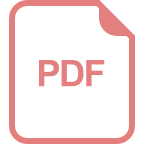














