boost库怎么判断JSON节点的类型
时间: 2023-11-16 20:07:10 浏览: 185
在boost库中,可以使用以下方法来判断JSON节点的类型:
1. 使用`is_null()`方法判断节点是否为null类型。
2. 使用`is_bool()`方法判断节点是否为bool类型。
3. 使用`is_number_integer()`方法判断节点是否为整数类型。
4. 使用`is_number_float()`方法判断节点是否为浮点数类型。
5. 使用`is_string()`方法判断节点是否为字符串类型。
6. 使用`is_array()`方法判断节点是否为数组类型。
7. 使用`is_object()`方法判断节点是否为对象类型。
例如,以下代码展示了如何使用boost库来判断JSON节点的类型:
```
#include <boost/property_tree/ptree.hpp>
#include <boost/property_tree/json_parser.hpp>
#include <iostream>
using boost::property_tree::ptree;
using boost::property_tree::read_json;
using boost::property_tree::write_json;
int main()
{
std::string json_str = "{\"name\":\"Alice\",\"age\":20,\"is_student\":true,\"marks\":[80,85,90]}";
ptree json_tree;
read_json(json_str, json_tree);
if (json_tree.get_child_optional("name"))
std::cout << "name: " << json_tree.get<std::string>("name") << std::endl;
if (json_tree.get_child_optional("age"))
std::cout << "age: " << json_tree.get<int>("age") << std::endl;
if (json_tree.get_child_optional("is_student"))
std::cout << "is_student: " << std::boolalpha << json_tree.get<bool>("is_student") << std::endl;
if (json_tree.get_child_optional("marks"))
{
ptree marks = json_tree.get_child("marks");
if (marks.size() > 0)
{
std::cout << "marks: ";
for (auto& mark : marks)
std::cout << mark.second.get_value<int>() << " ";
std::cout << std::endl;
}
}
if (json_tree.empty())
std::cout << "JSON is empty!" << std::endl;
return 0;
}
```
在上面的代码中,我们首先将一个JSON字符串解析为一个ptree对象,然后使用`get_child_optional()`方法获取节点,使用`get()`方法获取节点的值,并使用`is_null()`、`is_bool()`、`is_number_integer()`、`is_number_float()`、`is_string()`、`is_array()`、`is_object()`方法判断节点的类型。
阅读全文
相关推荐
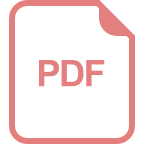
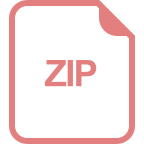
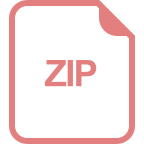












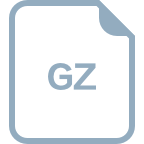
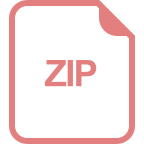
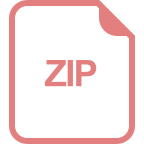