使用html-docx-js实现word下载并在分页中加表头
时间: 2024-01-06 09:07:11 浏览: 35
要使用html-docx-js实现word下载并在分页中加表头,可以按照以下步骤进行操作:
1. 安装html-docx-js
可以通过npm安装html-docx-js,命令如下:
```
npm install html-docx-js
```
也可以在浏览器中使用CDN引入html-docx-js。
2. 创建表格
可以使用HTML创建一个带有表头的表格,例如:
```html
<table>
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<tr>
<td>张三</td>
<td>18</td>
<td>男</td>
</tr>
<tr>
<td>李四</td>
<td>20</td>
<td>女</td>
</tr>
<tr>
<td>王五</td>
<td>22</td>
<td>男</td>
</tr>
</tbody>
</table>
```
3. 使用html-docx-js将表格转换为docx格式
可以使用html-docx-js将HTML中的内容转换为docx格式,例如:
```javascript
const htmlDocx = require('html-docx-js');
const tableHtml = `
<table>
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<tr>
<td>张三</td>
<td>18</td>
<td>男</td>
</tr>
<tr>
<td>李四</td>
<td>20</td>
<td>女</td>
</tr>
<tr>
<td>王五</td>
<td>22</td>
<td>男</td>
</tr>
</tbody>
</table>
`;
const docx = htmlDocx.asBlob(tableHtml);
// 下载docx文件
const downloadLink = document.createElement('a');
downloadLink.href = window.URL.createObjectURL(docx);
downloadLink.download = 'table.docx';
document.body.appendChild(downloadLink);
downloadLink.click();
```
4. 在分页中加表头
要在分页中加表头,可以将表头和表格分开,在表格上面放置一个固定位置的表头,例如:
```html
<div style="position: fixed; top: 0;">
<table>
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
</table>
</div>
<div style="margin-top: 60px;">
<table>
<tbody>
<tr>
<td>张三</td>
<td>18</td>
<td>男</td>
</tr>
<tr>
<td>李四</td>
<td>20</td>
<td>女</td>
</tr>
<tr>
<td>王五</td>
<td>22</td>
<td>男</td>
</tr>
<!-- 省略部分内容 -->
</tbody>
</table>
</div>
```
然后使用html-docx-js将两个部分分别转换为docx格式,最后合并成一个docx文件即可。
相关推荐
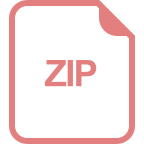
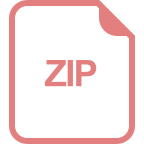














