条件:1.java微服务项目2.前端传入excel 3.excel数据包含多条数据4.数据内容:证件类型、证件号码。 需要实现:后端将前端传入的excel进行解析,转换成json格式的报文
时间: 2024-03-08 07:49:44 浏览: 162
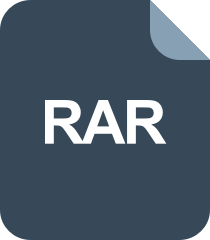
前端实现本地导入excel,在线解析预览,excel转json数据

好的。您可以使用Java中的Apache POI库来解析Excel文件。以下是一个简单的Java代码示例,演示如何使用Apache POI库将Excel文件解析为JSON格式的报文:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
import com.fasterxml.jackson.databind.ObjectMapper;
public class ExcelToJsonConverter {
public static void main(String[] args) throws IOException {
// 读取Excel文件
FileInputStream fileInputStream = new FileInputStream(new File("example.xlsx"));
Workbook workbook = WorkbookFactory.create(fileInputStream);
// 解析Excel文件
List<Customer> customers = new ArrayList<>();
Sheet sheet = workbook.getSheetAt(0);
Iterator<Row> rowIterator = sheet.rowIterator();
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
if (row.getRowNum() == 0) { // 跳过表头
continue;
}
Customer customer = new Customer();
customer.setDocumentType(row.getCell(0).getStringCellValue());
customer.setDocumentNumber(row.getCell(1).getStringCellValue());
customers.add(customer);
}
// 将解析结果转换为JSON格式的报文
ObjectMapper objectMapper = new ObjectMapper();
String json = objectMapper.writeValueAsString(customers);
System.out.println(json);
// 关闭资源
fileInputStream.close();
workbook.close();
}
public static class Customer {
private String documentType;
private String documentNumber;
public String getDocumentType() {
return documentType;
}
public void setDocumentType(String documentType) {
this.documentType = documentType;
}
public String getDocumentNumber() {
return documentNumber;
}
public void setDocumentNumber(String documentNumber) {
this.documentNumber = documentNumber;
}
}
}
```
在上面的代码中,我们首先使用`FileInputStream`类读取Excel文件,然后使用`WorkbookFactory`类将其转换为`Workbook`对象。接下来,我们遍历Excel文件中的每一行,将每一行中的证件类型和证件号码存储到`Customer`对象中。最后,我们使用`ObjectMapper`类将解析结果转换为JSON格式的报文。
需要注意的是,上面的代码仅仅是一个示例,您需要根据具体的业务需求进行修改。例如,如果Excel文件中包含多个表单,您需要通过`Workbook.getSheetAt()`方法获取指定的表单。另外,如果Excel文件中的数据类型不是文本类型,您需要根据实际情况调用`Cell`对象的不同方法来获取数据。
阅读全文
相关推荐
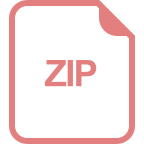
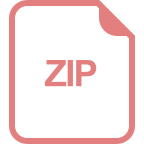
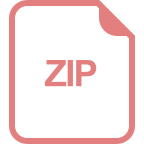
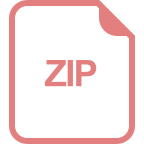
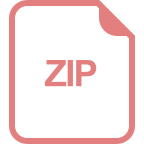
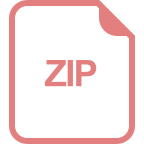
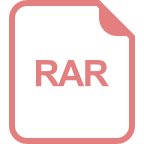
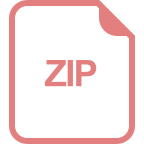
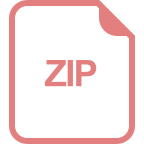
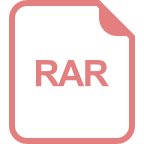
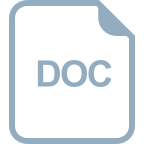
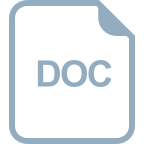
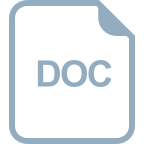
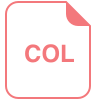
