pandas.concat
时间: 2023-10-12 07:09:22 浏览: 93
pandas.concat is a function in the pandas library that allows you to concatenate two or more pandas objects (DataFrames or Series) either vertically (along rows) or horizontally (along columns). It is similar to the SQL UNION operation.
Syntax:
``` python
pandas.concat(objs, axis=0, join='outer', ignore_index=False, keys=None, levels=None, names=None, verify_integrity=False, sort=False, copy=True)
```
Parameters:
- objs: a sequence or mapping of Series, DataFrame, or Panel objects to concatenate.
- axis: axis along which the objects will be concatenated. 0 for vertical concatenation and 1 for horizontal.
- join: type of join to be performed. Possible values are 'inner', 'outer', 'left', 'right'. The default is 'outer'.
- ignore_index: if True, the resulting object will have a new index. The default is False.
- keys: values to associate with objects being concatenated, forming a hierarchical index. Can either be a sequence or mapping.
- levels: specific levels (unique values) to use for constructing a MultiIndex. Otherwise, they will be inferred from the keys.
- names: names for the levels in the resulting hierarchical index.
- verify_integrity: if True, check if the new concatenated axis contains duplicates. If duplicates are found, raise a ValueError.
- sort: if True, sort the resulting axis.
- copy: if False, avoid copying data unnecessarily.
Returns:
A concatenated DataFrame or Series.
Example:
``` python
import pandas as pd
#creating two dataframes
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']})
df2 = pd.DataFrame({'A': ['A4', 'A5', 'A6', 'A7'],
'B': ['B4', 'B5', 'B6', 'B7'],
'C': ['C4', 'C5', 'C6', 'C7'],
'D': ['D4', 'D5', 'D6', 'D7']})
#concatenating the dataframes vertically
df = pd.concat([df1, df2], axis=0, ignore_index=True)
print(df)
```
Output:
```
A B C D
0 A0 B0 C0 D0
1 A1 B1 C1 D1
2 A2 B2 C2 D2
3 A3 B3 C3 D3
4 A4 B4 C4 D4
5 A5 B5 C5 D5
6 A6 B6 C6 D6
7 A7 B7 C7 D7
```
阅读全文
相关推荐
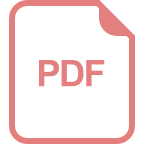
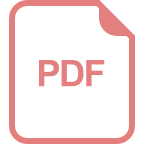
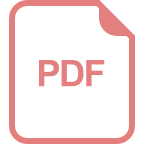












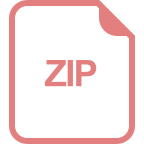
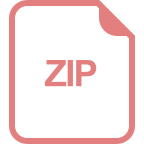