java 文件上传进度
时间: 2023-06-30 14:15:45 浏览: 98
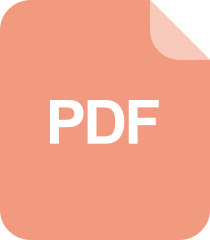
Java动态显示文件上传进度实现代码
要实现Java文件上传的进度监控,可以使用Java中的HttpURLConnection类和ProgressBar组件。以下是一个简单的实现步骤:
1. 使用HttpURLConnection类建立与服务器的连接,设置请求方法为POST,并设置文件上传参数。
2. 通过URLConnection获取输出流,将文件数据写入输出流中。
3. 在写入数据的过程中,使用ProgressBar组件更新进度条的进度。
4. 等待服务器响应,如果上传成功,则提示用户上传成功,否则提示上传失败。
以下是一个简单的示例代码,您可以根据实际情况进行修改和优化:
```java
import java.io.*;
import java.net.*;
import javax.swing.*;
public class FileUploader {
public static void main(String[] args) {
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(null);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
uploadFile(file);
} else {
JOptionPane.showMessageDialog(null, "请选择要上传的文件!");
}
}
private static void uploadFile(File file) {
String url = "http://localhost:8080/upload";
try {
URL uploadUrl = new URL(url);
HttpURLConnection connection = (HttpURLConnection) uploadUrl.openConnection();
connection.setRequestMethod("POST");
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type", "application/octet-stream");
connection.setRequestProperty("Content-Disposition", "attachment; filename=" + file.getName());
OutputStream outputStream = connection.getOutputStream();
BufferedInputStream inputStream = new BufferedInputStream(new FileInputStream(file));
byte[] buffer = new byte[1024];
int readLength = 0;
int totalLength = (int) file.length();
int uploadedLength = 0;
while ((readLength = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, readLength);
uploadedLength += readLength;
int progress = (int) (uploadedLength * 100 / totalLength);
System.out.println("已上传:" + progress + "%");
}
inputStream.close();
outputStream.flush();
outputStream.close();
if (connection.getResponseCode() == HttpURLConnection.HTTP_OK) {
JOptionPane.showMessageDialog(null, "上传成功!");
} else {
JOptionPane.showMessageDialog(null, "上传失败!");
}
} catch (Exception e) {
e.printStackTrace();
JOptionPane.showMessageDialog(null, "上传失败!");
}
}
}
```
在实际使用中,您需要将上述代码与GUI界面进行结合,以实现更好的用户体验。
阅读全文
相关推荐
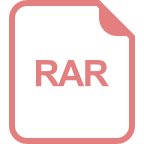
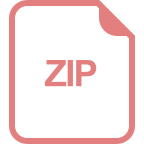















