java swing卡片布局
时间: 2023-09-08 20:17:14 浏览: 147
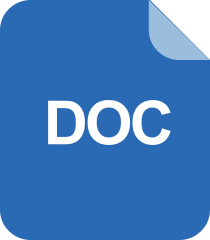
swing卡片布局
Java Swing提供了CardLayout类来实现卡片布局。卡片布局是一种容器,它允许在同一位置放置多个组件,并且只显示其中一个组件。用户可以通过操作各种控件来切换显示的组件。
以下是一个简单的示例程序,演示了如何使用卡片布局:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class CardLayoutDemo implements ActionListener {
JPanel cards; // a panel that uses CardLayout
final static String BUTTONPANEL = "Button Panel";
final static String TEXTPANEL = "Text Panel";
public void addComponentToPane(Container pane) {
// Put the JComboBox in a JPanel to get a nicer look.
JPanel comboBoxPane = new JPanel(); // use FlowLayout
String comboBoxItems[] = { BUTTONPANEL, TEXTPANEL };
JComboBox cb = new JComboBox(comboBoxItems);
cb.setEditable(false);
cb.addActionListener(this);
comboBoxPane.add(cb);
// Create the "cards".
JPanel card1 = new JPanel();
card1.add(new JButton("Button 1"));
card1.add(new JButton("Button 2"));
card1.add(new JButton("Button 3"));
JPanel card2 = new JPanel();
card2.add(new JTextField("TextField", 20));
// Create the panel that contains the "cards".
cards = new JPanel(new CardLayout());
cards.add(card1, BUTTONPANEL);
cards.add(card2, TEXTPANEL);
pane.add(comboBoxPane, BorderLayout.PAGE_START);
pane.add(cards, BorderLayout.CENTER);
}
public void actionPerformed(ActionEvent e) {
CardLayout cl = (CardLayout)(cards.getLayout());
cl.show(cards, (String)e.getSource());
}
private static void createAndShowGUI() {
// Create and set up the window.
JFrame frame = new JFrame("CardLayoutDemo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create and set up the content pane.
CardLayoutDemo demo = new CardLayoutDemo();
demo.addComponentToPane(frame.getContentPane());
// Display the window.
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
// Schedule a job for the event dispatch thread:
// creating and showing this application's GUI.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
createAndShowGUI();
}
});
}
}
```
在此示例中,我们使用了一个JComboBox来选择要显示的卡片。在addComponentToPane方法中,我们创建了两个卡片,一个包含三个按钮,另一个包含一个文本框。我们使用CardLayout将它们添加到一个面板中,然后将面板添加到主容器中。
在actionPerformed方法中,我们使用CardLayout的show方法来显示所选的卡片。
需要注意的是,CardLayout的show方法接受两个参数,第一个参数是包含卡片的面板,第二个参数是要显示的卡片的名称。在本例中,我们使用JComboBox的getSelectedItem方法获取所选卡片的名称,并将其作为第二个参数传递给show方法。
阅读全文
相关推荐
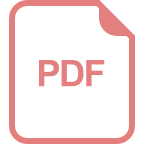



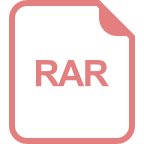
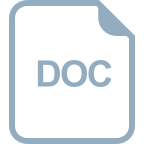
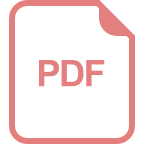
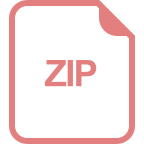
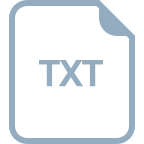
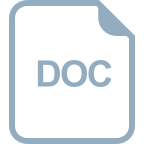
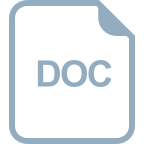
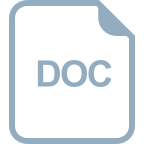
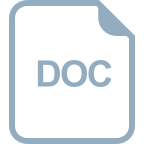
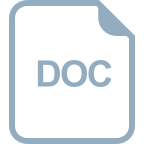
