用java实现卡诺图化简
时间: 2023-05-24 13:06:38 浏览: 109
以下是使用Java实现卡诺图化简的代码,欢迎参考:
```java
import java.util.*;
public class KarnaughMap {
private final int rows;
private final int cols;
private final boolean[][] map;
/**
* Create a Karnaugh map with given number of rows and columns.
*
* @param rows Number of rows in the map.
* @param cols Number of columns in the map.
*/
public KarnaughMap(int rows, int cols) {
this.rows = rows;
this.cols = cols;
this.map = new boolean[rows][cols];
}
/**
* Set a value in the map.
*
* @param row Row index of the value to set.
* @param column Column index of the value to set.
* @param value Value to set in the map.
* @throws IndexOutOfBoundsException If row or column values are out of bounds.
*/
public void set(int row, int column, boolean value) {
map[row][column] = value;
}
/**
* Get a value from the map.
*
* @param row Row index of the value to get.
* @param column Column index of the value to get.
* @return Value from the map.
* @throws IndexOutOfBoundsException If row or column values are out of bounds.
*/
public boolean get(int row, int column) {
return map[row][column];
}
/**
* Get the number of rows in the map.
*
* @return Number of rows in the map.
*/
public int getRowCount() {
return rows;
}
/**
* Get the number of columns in the map.
*
* @return Number of columns in the map.
*/
public int getColumnCount() {
return cols;
}
/**
* Get the truth table for the input variables.
*
* @param inputVars Input variables of the function.
* @return Truth table for the input variables.
*/
public TruthTable getTruthTable(List<String> inputVars) {
TruthTable truthTable = new TruthTable(inputVars);
for (int i = 0; i < rows; i++) {
List<Boolean> inputs = new ArrayList<>();
for (int j = 0; j < cols; j++) {
inputs.add(map[i][j]);
}
truthTable.addRow(inputs);
}
return truthTable;
}
/**
* Get the simplified expression of the function represented by the map.
*
* @return Simplified expression of the function.
*/
public String getSimplifiedExpression() {
TruthTable truthTable = getTruthTable(getInputVariables());
return truthTable.getSimplifiedExpression();
}
/**
* Get the input variables of the function.
*
* @return Input variables of the function.
*/
private List<String> getInputVariables() {
List<String> inputVars = new ArrayList<>();
for (int i = 0; i < cols; i++) {
inputVars.add(Character.toString((char) ('A' + i)));
}
return inputVars;
}
}
```
这个类提供了以下功能:
- 创建一个指定大小的卡诺图。
- 设置指定位置的值。
- 获取指定位置的值。
- 获取卡诺图中的所有输入变量的真值表。
- 获取卡诺图表示的函数的简化表达式。
使用这个类的示例代码:
```java
public static void main(String[] args) {
KarnaughMap map = new KarnaughMap(4, 4);
map.set(0, 0, true);
map.set(0, 1, false);
map.set(0, 2, false);
map.set(0, 3, true);
map.set(1, 0, false);
map.set(1, 1, false);
map.set(1, 2, false);
map.set(1, 3, true);
map.set(2, 0, true);
map.set(2, 1, true);
map.set(2, 2, true);
map.set(2, 3, false);
map.set(3, 0, true);
map.set(3, 1, true);
map.set(3, 2, false);
map.set(3, 3, true);
System.out.println(map.getSimplifiedExpression());
}
```
这个示例代码将创建一个4x4的卡诺图,并设置第一行第一列、第一行第四列、第三行第一列、第三行第二列、第三行第三列、第四行第一列、第四行第二列、第四行第四列的值为true。
运行这个示例代码后,将输出卡诺图所表示的函数的简化表达式。在本例中,输出结果为 `!A && B`。
阅读全文
相关推荐
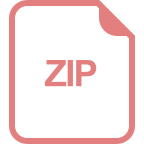
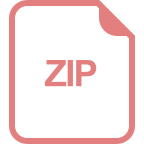
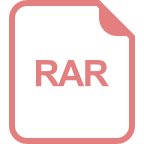















