c语言手写代码实现简单的copy和mv命令
时间: 2024-10-18 07:08:16 浏览: 24
在C语言中,手动编写复制文件(`cp`)和移动文件(`mv`)的简单版本通常涉及到文件操作和I/O处理。下面是一个非常基础的示例,展示了如何实现文件的复制:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE 1024
void copy_file(const char* src, const char* dst) {
FILE* src_file = fopen(src, "r");
if (src_file == NULL) {
perror("Error opening source file.");
return;
}
FILE* dst_file = fopen(dst, "w");
if (dst_file == NULL) {
perror("Error opening destination file.");
fclose(src_file);
return;
}
char buffer[MAX_LINE];
while (fgets(buffer, MAX_LINE, src_file)) {
fputs(buffer, dst_file);
}
fclose(src_file);
fclose(dst_file);
}
int main() {
copy_file("source.txt", "destination.txt");
printf("File copied successfully.\n");
return 0;
}
```
对于移动文件(`mv`),因为文件一旦移动就不可更改位置,所以这里可以先删除目标文件然后再进行复制:
```c
#include <stdbool.h>
bool remove_file(const char* filename) {
FILE* file = fopen(filename, "w");
fclose(file); // This will fail if the file exists, but we can use this for deletion check.
return remove(filename) == 0; // Use remove function to delete the file
}
void mv_file(const char* old_path, const char* new_path) {
if (!remove_file(new_path)) {
fprintf(stderr, "Error: File '%s' already exists.\n", new_path);
return;
}
copy_file(old_path, new_path);
printf("File moved successfully.\n");
}
int main() {
mv_file("old.txt", "new.txt");
return 0;
}
```
请注意,这仅是个简化的例子,在实际生产环境中,还需要考虑更多的错误处理和边界情况。此外,这个代码不会处理大文件,因为它一次性读取整个文件到内存中。
阅读全文
相关推荐
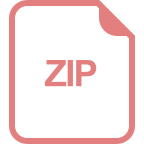
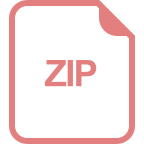
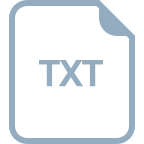
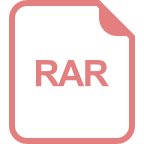
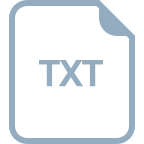
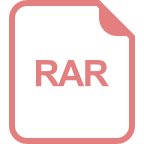
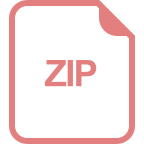
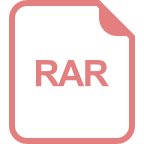