Spring Boot和Vue.js怎么实现文件打印功能
时间: 2024-05-02 20:16:12 浏览: 13
文件打印功能可以通过以下步骤实现:
1. 在Vue.js中,使用HTML5的File API将文件上传到服务器。
2. 在Spring Boot中,使用Apache POI或其他库将文件转换为可打印格式,例如PDF或DOCX。
3. 将转换后的文件返回给Vue.js,可以使用REST API或WebSocket等机制。
4. 在Vue.js中,使用JavaScript的打印API将文件打印出来。
具体实现步骤如下:
1. 在Vue.js中,使用以下代码上传文件:
```html
<template>
<div>
<input type="file" @change="handleFileUpload">
</div>
</template>
<script>
export default {
methods: {
handleFileUpload(event) {
const file = event.target.files[0]
const formData = new FormData()
formData.append('file', file)
// 发送文件到服务器
// ...
}
}
}
</script>
```
2. 在Spring Boot中,使用Apache POI将文件转换为PDF或DOCX格式,代码如下:
```java
// 导入Apache POI依赖
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
// 转换DOCX为PDF
public byte[] convertDocxToPdf(byte[] docxBytes) throws Exception {
try (XWPFDocument document = new XWPFDocument(new ByteArrayInputStream(docxBytes))) {
PdfOptions options = PdfOptions.create();
ByteArrayOutputStream out = new ByteArrayOutputStream();
PdfConverter.getInstance().convert(document, out, options);
return out.toByteArray();
}
}
// 转换XLSX为PDF
public byte[] convertXlsxToPdf(byte[] xlsxBytes) throws Exception {
try (XSSFWorkbook workbook = new XSSFWorkbook(new ByteArrayInputStream(xlsxBytes))) {
PdfOptions options = PdfOptions.create();
ByteArrayOutputStream out = new ByteArrayOutputStream();
PdfConverter.getInstance().convert(workbook, out, options);
return out.toByteArray();
}
}
```
3. 将转换后的文件返回给Vue.js,可以使用以下代码:
```java
@GetMapping("/download")
public ResponseEntity<?> downloadFile(@RequestParam String fileName) throws Exception {
byte[] fileBytes = getFileFromDatabase(fileName);
byte[] pdfBytes = convertFileToPdf(fileBytes);
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + fileName + ".pdf\"")
.contentType(MediaType.APPLICATION_PDF)
.body(pdfBytes);
}
```
4. 在Vue.js中,使用以下代码将文件打印出来:
```javascript
printFile() {
const iframe = document.createElement('iframe')
iframe.style.display = 'none'
iframe.src = '/api/download?fileName=' + this.fileName
iframe.onload = function() {
iframe.contentWindow.print()
document.body.removeChild(iframe)
}
document.body.appendChild(iframe)
}
```
相关推荐
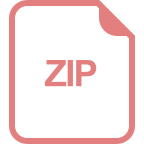
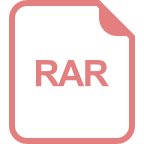
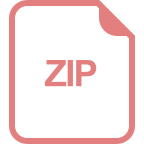














