用python做一个传奇界面
时间: 2024-02-02 13:03:27 浏览: 77
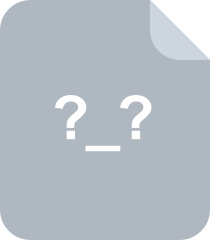
python界面例子
传奇界面是一个游戏界面,需要使用游戏引擎来实现,比较复杂。不过我们可以使用 Python 的 GUI 库来实现一个类似传奇的界面。这里以 tkinter 为例:
```python
import tkinter as tk
# 创建主窗口
root = tk.Tk()
root.title("传奇界面")
# 设置窗口大小和位置
width, height = 800, 600
screen_width, screen_height = root.winfo_screenwidth(), root.winfo_screenheight()
x, y = (screen_width - width) // 2, (screen_height - height) // 2
root.geometry("{}x{}+{}+{}".format(width, height, x, y))
# 创建背景图片
bg_image = tk.PhotoImage(file="bg.png")
bg_label = tk.Label(root, image=bg_image)
bg_label.place(x=0, y=0)
# 创建角色头像
head_image = tk.PhotoImage(file="head.png")
head_label = tk.Label(root, image=head_image)
head_label.place(x=50, y=50)
# 创建角色名字
name_label = tk.Label(root, text="传奇玩家", font=("宋体", 20))
name_label.place(x=150, y=60)
# 创建角色等级
level_label = tk.Label(root, text="等级:99", font=("宋体", 16))
level_label.place(x=150, y=120)
# 创建角色经验条
exp_label = tk.Label(root, text="经验:", font=("宋体", 16))
exp_label.place(x=150, y=150)
exp_canvas = tk.Canvas(root, width=200, height=20, bg="white")
exp_canvas.place(x=220, y=155)
exp_canvas.create_rectangle(0, 0, 200, 20, fill="lightgray")
exp_canvas.create_rectangle(0, 0, 150, 20, fill="green")
# 创建角色属性
str_label = tk.Label(root, text="力量:99", font=("宋体", 16))
str_label.place(x=500, y=120)
dex_label = tk.Label(root, text="敏捷:99", font=("宋体", 16))
dex_label.place(x=500, y=150)
int_label = tk.Label(root, text="智力:99", font=("宋体", 16))
int_label.place(x=500, y=180)
# 运行主窗口
root.mainloop()
```
这段代码会创建一个传奇界面的窗口,包括背景图片、角色头像、角色名字、角色等级、角色经验条、角色属性等元素。你需要用实际的图片替换代码中的 `bg.png` 和 `head.png`,并将其与代码放在同一目录下。
运行代码后,你将会看到一个类似传奇的界面。你可以根据自己的需求和想法,修改代码中的元素和布局,来实现你自己的传奇界面。
阅读全文
相关推荐








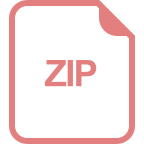
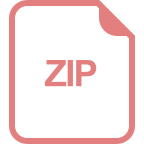
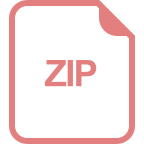
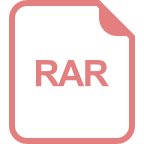
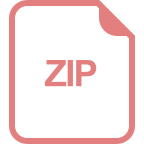
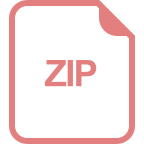
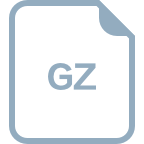
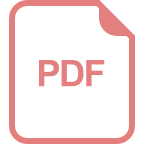