学生投票系统java代码javaEE
时间: 2024-03-20 07:36:57 浏览: 62
学生投票系统是一个常见的应用场景,下面是一个简单的学生投票系统的Java代码示例(使用JavaEE):
```java
// Student.java
public class Student {
private String name;
private int voteCount;
public Student(String name) {
this.name = name;
this.voteCount = 0;
}
public String getName() {
return name;
}
public int getVoteCount() {
return voteCount;
}
public void vote() {
voteCount++;
}
}
// VotingSystem.java
import java.util.ArrayList;
import java.util.List;
public class VotingSystem {
private List<Student> students;
public VotingSystem() {
this.students = new ArrayList<>();
}
public void addStudent(Student student) {
students.add(student);
}
public void vote(String studentName) {
for (Student student : students) {
if (student.getName().equals(studentName)) {
student.vote();
break;
}
}
}
public void printResults() {
for (Student student : students) {
System.out.println(student.getName() + ": " + student.getVoteCount());
}
}
}
// Main.java
public class Main {
public static void main(String[] args) {
VotingSystem votingSystem = new VotingSystem();
// 添加学生
Student student1 = new Student("Alice");
Student student2 = new Student("Bob");
votingSystem.addStudent(student1);
votingSystem.addStudent(student2);
// 进行投票
votingSystem.vote("Alice");
votingSystem.vote("Bob");
votingSystem.vote("Alice");
// 打印结果
votingSystem.printResults();
}
}
```
这个示例代码实现了一个简单的学生投票系统,包括学生类(Student)、投票系统类(VotingSystem)和主程序类(Main)。你可以根据实际需求进行扩展和修改。
阅读全文
相关推荐
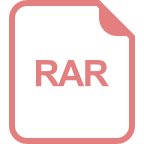
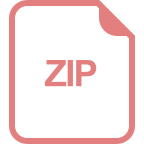
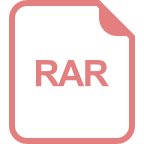
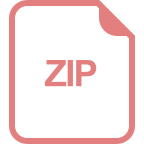
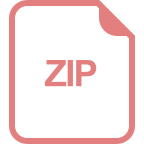
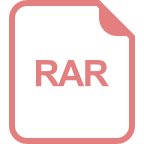
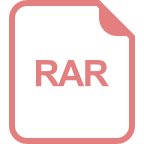
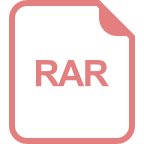
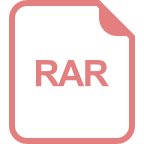
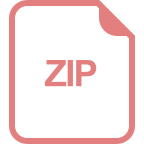
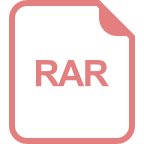
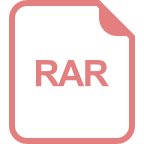
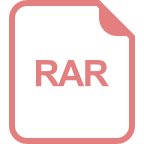
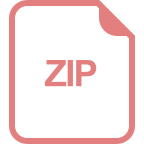