编写一个C语言程序,要求用户输入一个小写字母,然后输出该字母对应的大写字母,以及小写字母和大写字母分别对应的ASCII码值。
时间: 2024-10-12 15:15:59 浏览: 36
当你编写一个C语言程序来实现这个功能时,可以按照以下步骤进行:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char input;
// 请求用户输入一个小写字母
printf("请输入一个小写字母: ");
scanf("%c", &input);
// 检查是否输入的是小写字母
if (islower(input)) {
// 转换为大写字母
char upper = toupper(input);
// 输出转换后的字符和ASCII码值
printf("大写字母: %c\nASCII码(大写): %d\n", upper, (int)upper);
// 输出原小写字母和ASCII码值
printf("小写字母: %c\nASCII码(小写): %d\n", input, (int)input);
} else {
printf("这不是一个小写字母。\n");
}
return 0;
}
```
在这个程序中,我们首先导入了`stdio.h`用于输入输出,`ctype.h`包含了处理字符类型的函数如`islower()`和`toupper()`。用户输入一个字符后,如果它是一个小写字母,我们就将其转换为大写字母,并打印出原始和转换后的字符及其ASCII码值。如果不是小写字母,则会提示用户。
阅读全文
相关推荐
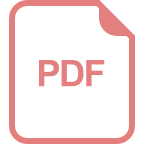
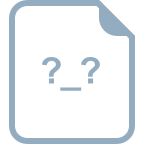















