java10 个评委给选手打分,分数在 1-100 之间,选手最后得分应该为去除最高分和最低分后的平均分。 编写程序实现:输入 10 个成绩,并获得这 10 个数中的最大值、最小值和总分,并且分数在1-100之间。
时间: 2024-04-19 20:29:40 浏览: 15
可以使用以下Java代码来实现这个功能:
```java
import java.util.Scanner;
public class ScoreCalculator {
public static void main(String[] args) {
int numOfJudges = 10;
int[] scores = new int[numOfJudges];
// 输入10个成绩
Scanner scanner = new Scanner(System.in);
for (int i = 0; i < numOfJudges; i++) {
System.out.print("请输入第" + (i + 1) + "个评委的分数:");
int score = scanner.nextInt();
// 判断分数是否在1-100之间
if (score < 1 || score > 100) {
System.out.println("分数应该在1-100之间,请重新输入!");
i--; // 重新输入该分数
continue;
}
scores[i] = score;
}
// 计算最大值、最小值和总分
int maxScore = scores[0];
int minScore = scores[0];
int totalScore = scores[0];
for (int i = 1; i < numOfJudges; i++) {
if (scores[i] > maxScore) {
maxScore = scores[i];
}
if (scores[i] < minScore) {
minScore = scores[i];
}
totalScore += scores[i];
}
// 去除最高分和最低分后的平均分
double averageScore = (totalScore - maxScore - minScore) / (numOfJudges - 2.0);
System.out.println("最高分:" + maxScore);
System.out.println("最低分:" + minScore);
System.out.println("总分:" + totalScore);
System.out.println("平均分:" + averageScore);
}
}
```
以上代码中,首先定义了评委人数 `numOfJudges` 和一个用于存储成绩的数组 `scores`。然后通过循环输入每个评委的分数,并进行范围判断。接着使用循环计算最大值、最小值和总分。最后根据公式计算去除最高分和最低分后的平均分,然后输出结果。
你可以运行这段代码并输入10个评委的分数,程序将会输出最高分、最低分、总分和平均分。
相关推荐








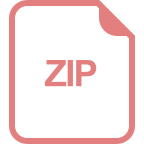