python图像分割大作业
时间: 2024-12-25 20:22:22 浏览: 10
### Python 图像分割大作业示例项目
#### 使用Matplotlib实现图像读取与预处理
为了完成图像分割的任务,首先需要能够有效地加载并显示目标图像。可以利用`matplotlib`库来简化这一流程。
```python
import matplotlib.pyplot as plt
from skimage import io
image_path = 'path_to_image'
img = io.imread(image_path)
plt.figure(figsize=(10, 8))
plt.imshow(img)
plt.axis('off')
plt.show()
```
这段代码展示了如何使用`skimage.io`模块中的函数读入图片,并借助于`matplotlib.pyplot`展示出来[^1]。
#### 基于随机森林的监督学习图像分割方案
对于更复杂的场景识别问题,则可能需要用到机器学习的方法来进行分类式的分割操作。这里介绍一种基于随机森林模型的技术路径:
```python
from sklearn.ensemble import RandomForestClassifier
import numpy as np
import cv2
def extract_features(image):
# 提取特征向量...
pass
# 加载训练数据集(假设已经准备好)
train_images = []
labels = []
for i in range(len(train_images)):
features = extract_features(train_images[i])
clf = RandomForestClassifier(n_estimators=100).fit(features_list, labels)
test_img = cv2.imread('new_test_image.jpg')
predicted_mask = clf.predict(extract_features(test_img))
cv2.imwrite('segmented_output.png', predicted_mask.astype(np.uint8)*255)
```
此部分说明了怎样构建一个简单的随机森林分类器用于预测新输入图像内的像素类别标签[^2]。
#### 利用OpenCV执行颜色空间转换及阈值化处理
当面对特定色彩模式下的对象分离需求时,转至HSV这样的感知均匀的颜色表示法往往能带来更好的效果;接着设定合理的上下限范围即可轻松获得理想的二进制掩模图层。
```python
hsv_img = cv2.cvtColor(cv2.imread('input_colorful_scene'), cv2.COLOR_BGR2HSV)
lower_blue = np.array([90, 50, 50])
upper_blue = np.array([130, 255, 255])
mask = cv2.inRange(hsv_img, lower_blue, upper_blue)
res = cv2.bitwise_and(hsv_img,hsv_img, mask= mask)
cv2.imshow('masked result', res)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
上述脚本实现了从BGR到HSV的空间映射以及针对蓝色调区域的选择性保留过程[^3]。
#### 结合形态学运算优化边缘检测后的输出质量
最后,在获取初步的结果之后还可以进一步采用诸如开闭运算之类的手段去改善边界处可能出现的一些瑕疵状况。
```python
kernel = np.ones((5,5),np.uint8)
opening = cv2.morphologyEx(mask,cv2.MORPH_OPEN,kernel, iterations = 2)
closing = cv2.morphologyEx(opening,cv2.MORPH_CLOSE,kernel,iterations = 2)
contours,_ = cv2.findContours(closing.copy(),cv2.RETR_EXTERNAL,cv2.CHAIN_APPROX_SIMPLE)[-2:]
final_result = cv2.drawContours(res.copy(), contours,-1,(0,255,0),thickness=cv2.FILLED)
cv2.imshow('Final Segmentation Result', final_result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
该片段演示了通过一系列形态学滤波增强最终呈现出来的清晰度和准确性[^4].
阅读全文
相关推荐
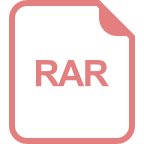
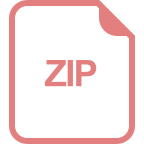
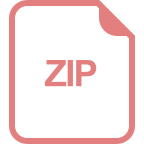
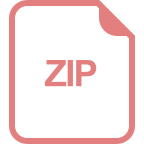
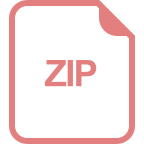
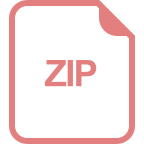
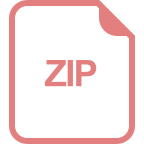
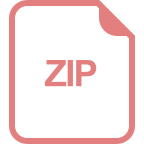
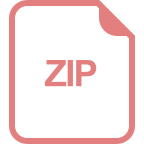
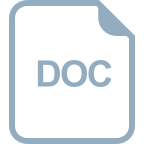
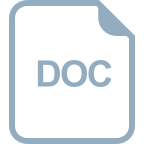
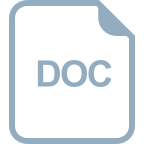
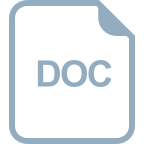
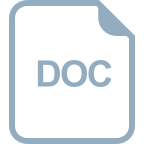