通讯录项目c语言代码(要有增删改查,顺序表是数组
时间: 2024-09-16 12:02:27 浏览: 56
在C语言中,我们可以使用结构体(struct)和动态数组(即数组指针)来实现一个简单的通讯录项目,包括增加、删除、修改和查询联系人功能。这里是一个基础示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_CONTACTS 10
typedef struct {
char name[50];
char phone_number[20];
} Contact;
Contact* contacts;
int contact_count = 0;
void add_contact(char* name, char* phone_number) {
if (contact_count >= MAX_CONTACTS) {
printf("通讯录已满,无法添加更多联系人。\n");
return;
}
Contact* new_contact = (Contact*) realloc(contacts, sizeof(Contact) * (contact_count + 1));
if (!new_contact) {
perror("内存分配失败");
exit(1);
}
new_contact[contact_count].name = name;
new_contact[contact_count].phone_number = phone_number;
contact_count++;
}
void delete_contact(int index) {
if (index < 0 || index >= contact_count) {
printf("无效索引,无法删除联系人。\n");
return;
}
memmove(&contacts[index], &contacts[index+1], sizeof(Contact) * (contact_count - index - 1));
contact_count--;
if (contact_count > 0)
contacts = realloc(contacts, sizeof(Contact) * contact_count);
else
free(contacts);
}
void modify_contact(int index, char* name, char* phone_number) {
if (index < 0 || index >= contact_count) {
printf("无效索引,无法修改联系人。\n");
return;
}
contacts[index].name = name;
contacts[index].phone_number = phone_number;
}
void search_contact(char* keyword) {
for (int i = 0; i < contact_count; i++) {
if (strstr(contacts[i].name, keyword) != NULL || strstr(contacts[i].phone_number, keyword) != NULL) {
printf("名称:%s, 电话:%s\n", contacts[i].name, contacts[i].phone_number);
}
}
}
int main() {
contacts = malloc(sizeof(Contact));
// 示例操作
add_contact("张三", "13812345678");
delete_contact(0);
modify_contact(1, "李四", "13987654321");
search_contact("三");
return 0;
}
```
在这个例子中,我们首先定义了一个`Contact`结构体表示每个联系人,并创建了动态数组`contacts`存储联系人信息。然后,通过一系列函数实现了对应的增删改查操作。
阅读全文
相关推荐
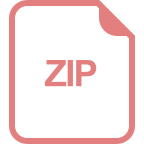
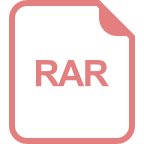
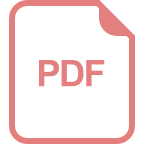















