qt做http服务端 怎么实现下载
时间: 2024-11-27 09:20:09 浏览: 11
在Qt中实现HTTP服务器并提供文件下载功能,可以利用Qt的网络框架,如QHttpServer模块。以下是基本步骤:
1. **设置环境**:
首先确保已安装Qt版本支持Web开发,并包含QHttpServer库。
2. **创建HTTP服务器**:
使用`QHttpServer::HttpRequestHandler`来处理每个请求。创建一个继承自这个处理器的类,例如`DownloadHandler`,用于处理下载请求。
```cpp
class DownloadHandler : public QHttpServer::HttpRequestHandler {
public:
DownloadHandler(QObject *parent = nullptr) : QHttpServer::HttpRequestHandler(parent) {}
protected:
void handleGet(const QString &uri) override {
// 检查URI是否表示一个文件路径
if (!uri.startsWith("/")) uri.prepend("/");
if (QFile::exists(server.rootPath() + uri)) {
QFile file(server.rootPath() + uri);
if (file.open(QIODevice::ReadOnly | QIODevice::ShareClose)) {
auto contentLength = file.size();
auto headers = QHttpResponseHeader().add("Content-Type", "application/octet-stream");
headers.add("Content-Disposition", "attachment; filename=" + file.fileName());
emit responseStarted(200, headers);
QByteArray data;
while (!file.atEnd()) {
data.append(file.read(1024));
emit bytesWritten(data.size());
}
file.close();
emit finished();
} else {
emit error(QAbstractSocket::HostNotFoundError, tr("File not found"));
}
} else {
emit error(QAbstractSocket::NetworkError, tr("Invalid URI"));
}
}
};
```
3. **启动服务器**:
在主应用循环中,初始化服务器,并注册下载处理器。
```cpp
QCoreApplication app(argc, argv);
QHttpServer server(QStringLiteral("YourServerName"), &app);
server.setRootPath(QStringLiteral("path/to/files")); // 设置服务器文件根目录
DownloadHandler downloadHandler;
server.registerRequestHandler(downloadHandler);
server.listen(QHostAddress::Any, 8080); // 监听任意地址的8080端口
app.exec();
```
阅读全文
相关推荐
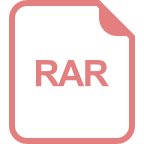
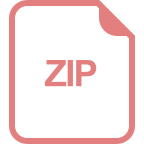
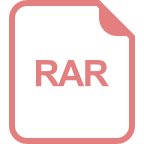
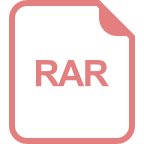
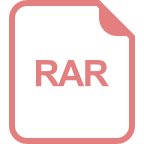
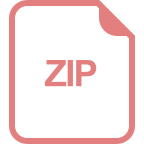
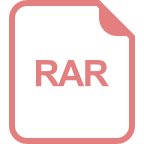
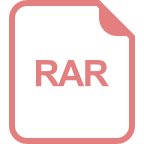
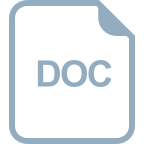







