Qt打开指定目录并选中文件 多种方法
时间: 2024-09-14 13:14:09 浏览: 97
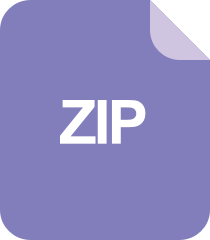
Qt目录树制作
在Qt框架中,打开指定目录并选中文件可以通过多种方法实现,以下是一些常见的方法:
1. 使用`QFileDialog`类:
`QFileDialog`是Qt中用于打开和保存文件的对话框组件。通过设置`QFileDialog`的`directory`属性来指定目录,并通过设置`selectedFiles`属性来选中特定的文件。
```cpp
#include <QFileDialog>
#include <QPushButton>
QPushButton *button = new QPushButton("打开文件夹并选中文件");
connect(button, &QPushButton::clicked, this, [this]() {
QString directory = "/path/to/directory";
QString fileName = "/path/to/directory/selectedFile.txt";
QFileDialog dialog(this);
dialog.setDirectory(directory);
dialog.selectFile(fileName);
dialog.setFileMode(QFileDialog::ExistingFile);
dialog.exec();
});
```
2. 使用`QDesktopServices`和`QUrl`:
如果你的目标是仅仅让系统打开一个文件夹而不直接在Qt应用程序中显示,可以使用`QDesktopServices`和`QUrl`。
```cpp
#include <QDesktopServices>
#include <QUrl>
QString folderPath = "/path/to/directory";
QDesktopServices::openUrl(QUrl::fromLocalFile(folderPath));
```
3. 使用`QProcess`启动系统关联的应用程序:
如果你想通过系统默认的文件管理器打开一个文件夹,并且选中其中的文件,可以使用`QProcess`启动与文件夹关联的默认程序。
```cpp
#include <QProcess>
QString folderPath = "/path/to/directory";
QString command = "explorer"; // Windows系统使用"explorer"
QStringList arguments;
arguments << "/select," << folderPath; // Windows系统使用"/select,"来选中文件
QProcess::startDetached(command, arguments);
```
4. 使用系统调用(仅限于某些操作系统):
你可以通过C++的`system`函数或者Qt的`QProcess`来执行系统命令,例如在Windows上使用`explorer`命令。
```cpp
#include <QProcess>
QString folderPath = "/path/to/directory";
QProcess::execute(QString("explorer /select,\"%1\"").arg(folderPath));
```
注意:使用`QDesktopServices`和系统调用可能会依赖于特定的操作系统,因此在跨平台应用中需要特别注意。
阅读全文
相关推荐
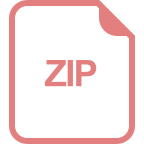
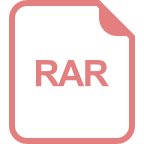
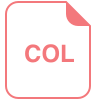
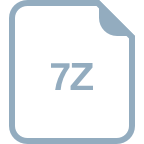
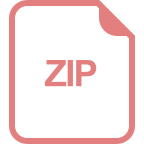
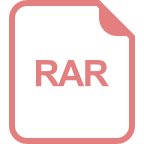
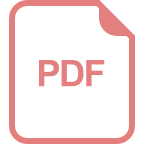
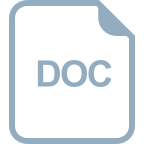
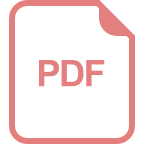
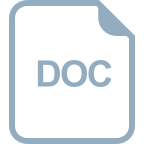
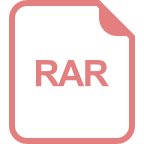
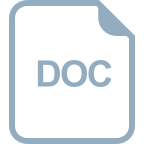
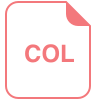
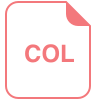
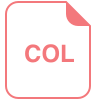
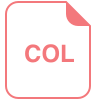
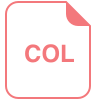
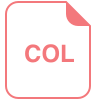