多线程 调度 实现代码 C++
时间: 2024-04-18 18:17:17 浏览: 11
以下是一个简单的多线程调度实现代码,使用了 C++11 的线程库:
```c++
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
using namespace std;
mutex mtx;
condition_variable cv;
bool ready = false;
void worker(int id) {
unique_lock<mutex> lk(mtx);
while (!ready) {
cv.wait(lk);
}
cout << "Worker " << id << " is working..." << endl;
}
int main() {
const int num_workers = 5;
thread workers[num_workers];
for (int i = 0; i < num_workers; i++) {
workers[i] = thread(worker, i);
}
// signal all workers to start working
{
lock_guard<mutex> lk(mtx);
ready = true;
}
cv.notify_all();
for (int i = 0; i < num_workers; i++) {
workers[i].join();
}
return 0;
}
```
这个例子中有 5 个 worker 线程,它们需要等待主线程发出信号后才开始工作。主线程通过 `condition_variable` 和 `mutex` 来实现等待和通知的机制。主线程先创建 5 个 worker 线程,然后将 `ready` 变量设置为 true,并发出通知信号。worker 线程在等待 `ready` 变量的值变为 true 时被唤醒,并开始工作。在这个例子中,所有 worker 线程都会同时开始工作,但实际上可以通过控制 `ready` 变量的值来实现更复杂的调度逻辑。
相关推荐
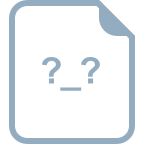














