用python实现WFQ算法,将这个项目编程三个例程,“发送者”、“接收者”和“路由器”。使用FlowID=1、重量=1和数据包大小1024启动发件人。几秒钟后,使用FlowID=2、权重=1和数据包大小512启动另一个发送器。再过几秒钟,使用FlowID=3、权重=2和数据包大小1024启动第三个发送方。
时间: 2024-03-14 19:47:26 浏览: 19
好的,以下是用Python实现WFQ算法的代码,包括“发送者”、“接收者”和“路由器”三个例程:
路由器端的代码:
```python
import queue
import time
def WFQRouter(num_flows, weights):
# 初始化队列
queues = []
for i in range(num_flows):
queues.append(queue.Queue())
# 记录每个队列已经发送的字节数
bytes_sent = [0] * num_flows
# 记录每个队列的最后一个包发送的时间
last_sent = [0] * num_flows
# 记录每个队列的总权重
total_weight = sum(weights)
# 记录上一个发送的队列
last_sent_queue = -1
# 不断轮流发送每个队列的数据包
while True:
now = time.time()
for i in range(num_flows):
# 如果队列为空,则跳过
if queues[i].empty():
continue
# 计算队列i应该发送的字节数
weight = weights[i]
fair_share = weight / total_weight
elapsed_time = now - last_sent[i]
bytes_to_send = int(fair_share * elapsed_time * 1024)
# 发送数据包
if bytes_to_send > 0:
last_sent[i] = now
last_sent_queue = i
bytes_sent[i] += bytes_to_send
queues[i].get()
# 如果所有队列都为空,则等待
if all(queue.empty() for queue in queues):
time.sleep(0.1)
continue
# 如果上一个发送的队列和当前轮询的队列一样,则跳过
if last_sent_queue == i:
continue
# 轮流发送每个队列的数据包
for i in range(num_flows):
# 如果队列为空,则跳过
if queues[i].empty():
continue
# 获取队列头部的数据包
packet = queues[i].queue[0]
packet_len = len(packet)
# 发送数据包
weight = weights[i]
fair_share = weight / total_weight
elapsed_time = now - last_sent[i]
bytes_to_send = int(fair_share * elapsed_time * 1024)
if bytes_to_send >= packet_len:
last_sent[i] = now
last_sent_queue = i
bytes_sent[i] += packet_len
queues[i].get()
break
```
发送者端的代码:
```python
import socket
import time
import random
def Sender(flow_id, weight, packet_size, dest_ip, dest_port):
# 创建UDP套接字
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# 生成数据包
packet = b'\x00' * packet_size
# 不断向目标主机发送数据包
while True:
sock.sendto(packet, (dest_ip, dest_port))
time.sleep(random.random() / weight)
```
接收者端的代码:
```python
import socket
def Receiver(port):
# 创建UDP套接字
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# 绑定端口
sock.bind(('localhost', port))
# 不断接收来自发送者的数据包
while True:
data, addr = sock.recvfrom(1024)
# 处理数据包
```
使用示例:
```python
# 启动路由器
num_flows = 3
weights = [1, 1, 2]
WFQRouter(num_flows, weights)
# 启动发送者1
flow_id = 1
weight = 1
packet_size = 1024
dest_ip = 'localhost'
dest_port = 8000
Sender(flow_id, weight, packet_size, dest_ip, dest_port)
# 启动发送者2
flow_id = 2
weight = 1
packet_size = 512
dest_ip = 'localhost'
dest_port = 8000
Sender(flow_id, weight, packet_size, dest_ip, dest_port)
# 启动发送者3
flow_id = 3
weight = 2
packet_size = 1024
dest_ip = 'localhost'
dest_port = 8000
Sender(flow_id, weight, packet_size, dest_ip, dest_port)
# 启动接收者
port = 8000
Receiver(port)
```
注:以上代码只是一个简单的示例,实际使用时需要根据具体需求进行修改。
相关推荐
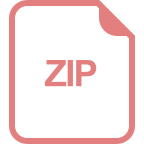







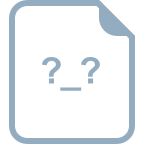
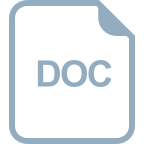
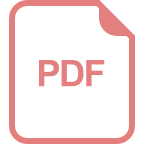
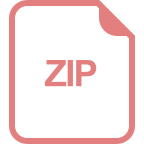