请你扮演一个资深前端,用vue2+百度地图api+element写一个地图选点(通过弹框形式)并且可搜索地点,最后选择的功能,写一个完整案例
时间: 2024-02-27 22:55:24 浏览: 42
好的,我会尽力回答你的问题。首先,我们需要引入 Vue、Element UI 和百度地图 API 的相关文件。
```html
<!-- 引入 Vue -->
<script src="https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.min.js"></script>
<!-- 引入 Element UI -->
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
<script src="https://unpkg.com/element-ui/lib/index.js"></script>
<!-- 引入百度地图 API -->
<script src="http://api.map.baidu.com/api?v=3.0&ak=your_ak"></script>
```
在 HTML 中,我们需要创建一个地图容器和一个按钮,用于触发弹框。
```html
<div id="app">
<div id="map"></div>
<el-button type="primary" @click="showDialog">选择位置</el-button>
</div>
```
在 Vue 实例中,我们需要创建一个地图对象,并且在弹框中显示一个搜索框和地图。
```javascript
new Vue({
el: '#app',
data: {
map: null, // 地图对象
point: null, // 选中的点
dialogVisible: false, // 弹框可见性
searchValue: '', // 搜索框的值
searchResult: [] // 搜索结果
},
mounted() {
// 创建地图对象
this.map = new BMap.Map("map");
this.map.centerAndZoom(new BMap.Point(116.404, 39.915), 11);
this.map.enableScrollWheelZoom(true);
},
methods: {
showDialog() {
// 显示弹框
this.dialogVisible = true;
// 创建搜索框和地图
const searchBox = new BMapLib.SearchBox("searchBox", {
onSearchComplete: (results) => {
// 处理搜索结果
this.searchResult = results.map((result) => {
return {
title: result.title,
address: result.address,
point: result.point
};
});
}
});
const map = new BMap.Map("dialogMap");
const point = this.point || new BMap.Point(116.404, 39.915);
map.centerAndZoom(point, 15);
map.enableScrollWheelZoom(true);
const marker = new BMap.Marker(point);
map.addOverlay(marker);
// 监听搜索结果的点击事件
map.addEventListener("click", (e) => {
marker.setPosition(e.point);
this.point = e.point;
});
},
handleSelect(item) {
// 选择一个点
this.point = item.point;
this.dialogVisible = false;
}
}
});
```
在弹框中,我们需要显示一个搜索框和地图,以及搜索结果列表。当用户输入关键词并点击搜索时,我们需要使用百度地图的搜索服务来获取搜索结果。当用户选择一个搜索结果时,我们需要在地图上显示该点,并且将其作为选择的点。
```html
<el-dialog :visible.sync="dialogVisible" width="70%" title="选择位置">
<el-input placeholder="请输入关键词" v-model="searchValue" @keydown.enter.native="search"></el-input>
<div id="dialogMap" style="height: 400px;"></div>
<div id="searchBox"></div>
<el-table :data="searchResult" @row-click="handleSelect">
<el-table-column prop="title" label="名称"></el-table-column>
<el-table-column prop="address" label="地址"></el-table-column>
</el-table>
</el-dialog>
```
```javascript
search() {
// 搜索关键词
const searchBox = new BMapLib.SearchBox("searchBox", {
onSearchComplete: (results) => {
// 处理搜索结果
this.searchResult = results.map((result) => {
return {
title: result.title,
address: result.address,
point: result.point
};
});
}
});
searchBox.search(this.searchValue);
}
```
以上就是一个完整的地图选点的案例,你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
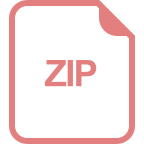













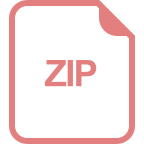
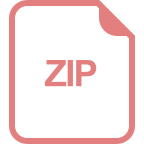