vue实现canvas弧形滑块
时间: 2023-09-06 15:10:17 浏览: 171
要实现一个canvas弧形滑块,可以按照以下步骤进行:
1. 引入vue和canvas,在vue组件中创建一个canvas元素。
2. 通过canvas API绘制一个弧形,可以使用arc方法绘制圆弧。
3. 绘制滑块,可以使用fillRect方法绘制一个矩形。
4. 监听鼠标事件(mousedown、mousemove、mouseup),根据鼠标位置计算滑块的位置,并重新绘制canvas。
下面是一个简单的实现代码:
```html
<template>
<canvas ref="canvas"></canvas>
</template>
<script>
export default {
mounted() {
const canvas = this.$refs.canvas
const ctx = canvas.getContext('2d')
const width = canvas.width
const height = canvas.height
const centerX = width / 2
const centerY = height / 2
const radius = 100
const startAngle = Math.PI * 1.5
const endAngle = Math.PI * 2.5
let position = 0
let dragging = false
function draw() {
// 绘制弧形
ctx.beginPath()
ctx.arc(centerX, centerY, radius, startAngle, endAngle)
ctx.lineWidth = 10
ctx.strokeStyle = '#ddd'
ctx.stroke()
// 绘制滑块
const sliderWidth = 20
const sliderHeight = 40
const x = centerX + Math.cos(position) * radius - sliderWidth / 2
const y = centerY + Math.sin(position) * radius - sliderHeight / 2
ctx.fillStyle = '#aaa'
ctx.fillRect(x, y, sliderWidth, sliderHeight)
}
function getPosition(x, y) {
const dx = x - centerX
const dy = y - centerY
return Math.atan2(dy, dx)
}
function handleMouseDown(e) {
const x = e.clientX - canvas.offsetLeft
const y = e.clientY - canvas.offsetTop
const distance = Math.sqrt(Math.pow(centerX - x, 2) + Math.pow(centerY - y, 2))
if (distance > radius - 10 && distance < radius + 10) {
dragging = true
}
}
function handleMouseMove(e) {
if (dragging) {
const x = e.clientX - canvas.offsetLeft
const y = e.clientY - canvas.offsetTop
position = getPosition(x, y)
draw()
}
}
function handleMouseUp() {
dragging = false
}
draw()
canvas.addEventListener('mousedown', handleMouseDown)
canvas.addEventListener('mousemove', handleMouseMove)
canvas.addEventListener('mouseup', handleMouseUp)
}
}
</script>
```
以上代码创建了一个canvas元素,并在mounted钩子函数中绘制了一个弧形和一个滑块。同时,监听了鼠标事件,在鼠标拖动时计算滑块的位置并重新绘制canvas。
阅读全文
相关推荐
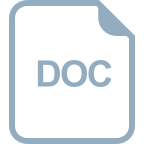
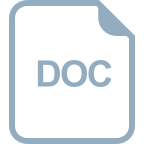
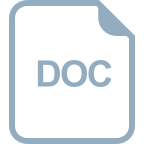
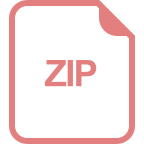
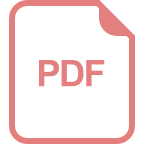
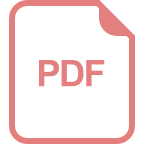
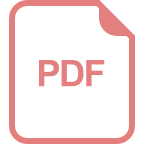
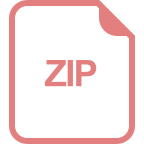
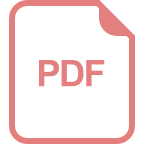
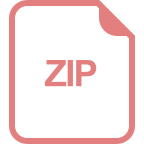
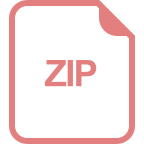
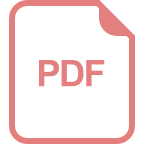
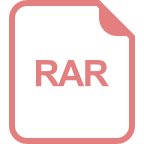
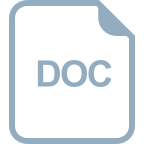
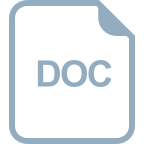
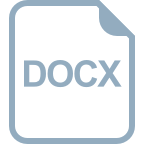
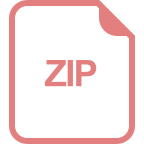