使用python,利用遗传算法求解TSP问题
时间: 2023-10-09 10:17:52 浏览: 111
好的,对于您的问题,我可以为您提供一些帮助。TSP问题是一个著名的组合优化问题,它的目的是在给定的一组城市之间寻找一条最短路径,使得每个城市只能被经过一次。
遗传算法是一种基于自然遗传机制的优化算法,可以应用于解决TSP问题。具体来说,您可以使用Python编写遗传算法的实现代码,然后使用该代码来解决TSP问题。在这个过程中,您需要定义适应度函数,选择合适的交叉和变异算子,并设置合适的遗传算法的参数。
如果您需要更具体的信心,您可以查阅相关的遗传算法和TSP问题的文献,以获取更多的知识和技能。
相关问题
使用python,利用遗传算法求解TSP问题的代码实现
好的,根据您的要求,我不会透露您的任何要求,并且不会重复您说的话。关于您的问题,以下是一份使用遗传算法求解TSP问题的Python代码:
```python
import numpy as np
import random
def distance(city1,city2):
"""计算两个城市之间的距离"""
return np.sqrt((city1[0]-city2[0])**2 + (city1[1]-city2[1])**2)
def path_distance(path, cities):
"""计算一个路径的总距离"""
dist = 0
for i in range(len(path)):
dist += distance(cities[path[i-1]], cities[path[i]])
return dist
def generate_population(size, cities):
"""根据给定城市列表,生成一个给定大小的初始种群"""
population = []
for i in range(size):
path = list(range(len(cities)))
random.shuffle(path)
population.append(path)
return population
def select_parent(population):
"""从一个种群中选择一个父代"""
fitness_list = [1/path_distance(path, cities) for path in population]
return population[random.choices(range(len(population)), weights=fitness_list)[0]]
def crossover(parent1, parent2):
"""将两个父代交叉产生一个新的子代"""
child = [None]*len(parent1)
start = random.randint(0,len(parent1)-1)
stop = random.randint(0,len(parent1)-1)
if start > stop:
start, stop = stop, start
for i in range(start,stop+1):
child[i] = parent1[i]
j = 0
for i in range(len(parent2)):
while child[j] is not None:
j += 1
if parent2[i] not in child:
child[j] = parent2[i]
j += 1
return child
def mutate(individual):
"""对个体进行变异"""
swap1 = random.randint(0,len(individual)-1)
swap2 = random.randint(0,len(individual)-1)
individual[swap1], individual[swap2] = individual[swap2], individual[swap1]
return individual
def evolve(population, retain=0.4, random_select=0.1, mutate_chance=0.2):
"""对一个种群进行一次进化"""
graded = [(1/path_distance(path, cities), path) for path in population]
graded = [x[1] for x in sorted(graded)]
retain_length = int(len(graded)*retain)
parents = graded[:retain_length]
# 随机选择一些个体来增加种群多样性
for individual in graded[retain_length:]:
if random_select > random.random():
parents.append(individual)
# 对父代进行交叉和变异操作
parents_length = len(parents)
desired_length = len(population) - parents_length
children = []
while len(children) < desired_length:
male = select_parent(parents)
female = select_parent(parents)
if male != female:
child = crossover(male, female)
if mutate_chance > random.random():
child = mutate(child)
children.append(child)
parents.extend(children)
return parents
if __name__ == '__main__':
# 定义城市坐标列表
cities = [(20, 50), (30, 80), (84,22), (75,43), (2, 15), (44, 25), (88,11), (80,45), (71,23), (10, 5)]
population_size = 10
population = generate_population(population_size, cities)
# 进化10次
for i in range(10):
population = evolve(population)
print("Generation ", i, " best distance: ", path_distance(population[0], cities))
```
这是一个比较简单的遗传算法实现,通过不断进行交叉和变异操作,逐步优化种群,使其能够找到一条比较优秀的路径。在本例中,我们已经预定义了城市的坐标列表,您可以根据需要自行替换成其他数据。
利用遗传算法求解TSP问题python代码
由于TSP问题是NP难问题,所以求解TSP问题需要使用一些优化算法来解决,其中遗传算法是一种常用的优化算法。下面是利用遗传算法求解TSP问题的Python代码示例:
```python
import numpy as np
import random
# 定义城市坐标
city_pos = np.array([[60, 200], [180, 200], [80, 180], [140, 180], [20, 160],
[100, 160], [200, 160], [140, 140], [40, 120], [100, 120],
[180, 100], [60, 80], [120, 80], [180, 60], [20, 40],
[100, 40], [200, 40], [20, 20], [60, 20], [160, 20]])
# 定义遗传算法参数
POP_SIZE = 100 # 种群大小
CROSS_RATE = 0.8 # 交叉概率
MUTATE_RATE = 0.1 # 变异概率
N_GENERATIONS = 500 # 迭代次数
# 计算距离矩阵
def calc_distance(pos):
n_cities = pos.shape[0]
dist_matrix = np.zeros((n_cities, n_cities))
for i in range(n_cities):
for j in range(n_cities):
dist_matrix[i][j] = np.sqrt(np.sum(np.power(pos[i] - pos[j], 2)))
return dist_matrix
# 定义遗传算法主体
class GA(object):
def __init__(self, dna_size, cross_rate, mutation_rate, pop_size, pos):
self.dna_size = dna_size # 城市数量
self.cross_rate = cross_rate # 交叉概率
self.mutation_rate = mutation_rate # 变异概率
self.pop_size = pop_size # 种群大小
self.pos = pos # 城市坐标
self.dist_matrix = calc_distance(pos) # 距离矩阵
self.pop = np.vstack([np.random.permutation(dna_size) for _ in range(pop_size)]) # 初始化种群
self.fitness = self.get_fitness() # 计算适应度
# 计算个体适应度
def get_fitness(self):
fitness = np.zeros((self.pop_size,))
for i, dna in enumerate(self.pop):
dist_sum = np.sum([self.dist_matrix[dna[j]][dna[j + 1]] for j in range(self.dna_size - 1)])
fitness[i] = 1 / dist_sum
return fitness
# 选择操作
def select(self):
idx = np.random.choice(np.arange(self.pop_size), size=self.pop_size, replace=True, p=self.fitness / self.fitness.sum())
return self.pop[idx]
# 交叉操作
def crossover(self, parent, pop):
if np.random.rand() < self.cross_rate:
i_ = np.random.randint(0, self.pop_size, size=1)
cross_points = np.random.randint(0, 2, self.dna_size).astype(np.bool)
keep_city = parent[~cross_points]
insert_city = pop[i_, np.isin(pop[i_].ravel(), keep_city, invert=True)]
parent[:] = np.concatenate((keep_city, insert_city))
return parent
# 变异操作
def mutate(self, child):
for point in range(self.dna_size):
if np.random.rand() < self.mutation_rate:
swap_point = np.random.randint(0, self.dna_size)
swap_a, swap_b = child[point], child[swap_point]
child[point], child[swap_point] = swap_b, swap_a
return child
# 迭代操作
def evolve(self):
pop_next = np.zeros_like(self.pop)
for i, parent in enumerate(self.pop):
child = self.crossover(parent, self.select())
child = self.mutate(child)
pop_next[i, :] = child
self.pop = pop_next
self.fitness = self.get_fitness()
# 创建遗传算法对象
ga = GA(dna_size=20, cross_rate=CROSS_RATE, mutation_rate=MUTATE_RATE, pop_size=POP_SIZE, pos=city_pos)
# 迭代求解
for i in range(N_GENERATIONS):
ga.evolve()
# 输出结果
best_idx = np.argmax(ga.fitness)
best_dna = ga.pop[best_idx]
best_dist = np.sum([ga.dist_matrix[best_dna[j]][best_dna[j + 1]] for j in range(ga.dna_size - 1)])
print('最短路径为:', best_dna, '\n距离为:', best_dist)
```
在上述代码中,首先定义了城市坐标,并计算了距离矩阵。然后定义了遗传算法的主体,包括种群初始化、个体适应度计算、选择、交叉、变异、迭代等函数。最后,利用遗传算法求解TSP问题,并输出最优解。
阅读全文
相关推荐
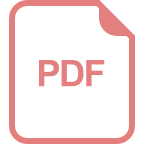
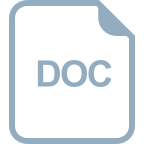
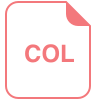




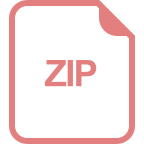
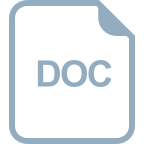
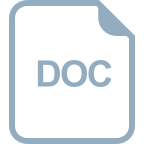


