俄罗斯方块初始界面c语言代码
时间: 2024-09-30 20:10:38 浏览: 32
要创建一个基本的俄罗斯方块游戏的初始界面,我们需要考虑以下几个关键元素:窗口初始化、标题设置、以及基本的图形绘制。由于你是请求用C语言编写,这里我会提供一个简单的控制台版本的示例,因为使用C库如ncurses或SDL在Windows上可能更复杂。
```c
#include <stdio.h>
#include <conio.h> // Windows console input functions
// Function prototypes
void draw_board(int board[4][6]); // Draw the game board
void display_message(char *msg); // Display a message
int main() {
int board[4][6] = {0}; // Initialize an empty board
char msg[50] = "Welcome to Tetris! Press any key to start..."; // Initial message
bool playing = false; // Flag to indicate if game is running
while (true) {
// Check for user input to start the game or exit
if (_kbhit()) {
playing = true;
break;
}
display_message(msg);
draw_board(board);
}
if (playing) {
printf("Starting game...\n");
// Game logic goes here, which you'll need to implement separately
} else {
printf("Exiting...\n");
}
return 0;
}
// Draw the board function, this is a placeholder and would need to be expanded
void draw_board(int board[4][6]) {
for (int i = 0; i < 4; ++i) {
for (int j = 0; j < 6; ++j) {
// You can use ASCII characters to represent blocks on the board
printf("%c ", board[i][j]);
}
printf("\n");
}
}
// Display a message function, also a placeholder
void display_message(char *msg) {
printf("%s\n", msg);
}
```
这个代码只是一个起点,实际的游戏逻辑(例如旋转、下落和消除行)需要你自己去实现。而且,为了创建一个完整的图形化的界面,你可能需要使用像`ncurses`这样的库,而不是标准的`printf`和`_kbhit()`函数。
阅读全文
相关推荐
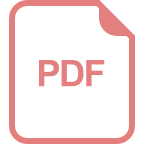
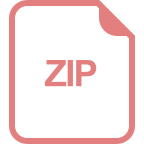
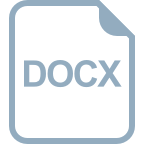
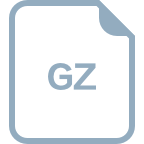
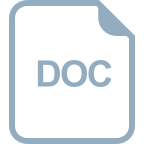
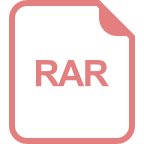
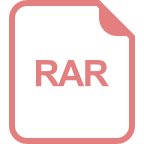
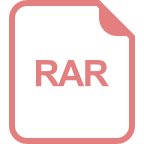
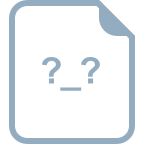
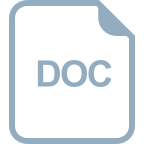
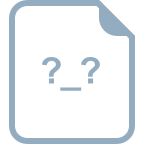
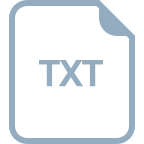
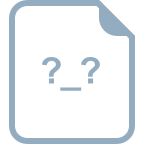
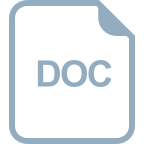
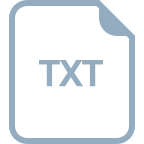
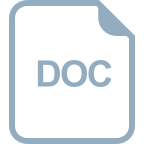
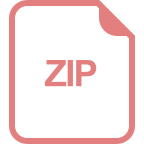