Char 9: runtime error: reference binding to null pointer of type 'std::vector<int, std::allocator<int>>' (stl_vector.h) SUMMARY: UndefinedBehaviorSanitizer: undefined-behavior /usr/bin/../lib/gcc/x86_64-linux-gnu/9/../../../../include/c++/9/bits/stl_vector.h:1043:9
时间: 2023-07-19 19:49:48 浏览: 844
这个错误发生在试图将一个空指针赋值给vector的引用或指针时。原因可能是你没有正确地初始化vector或者在使用vector之前已经将其销毁了。以下是可能的解决方案:
- 确保在使用vector之前对其进行了正确的初始化,例如使用vector的构造函数或push_back方法添加元素。
- 检查你的代码是否错误地将vector销毁了,例如在其它函数中删除了vector或使用了已经销毁的vector。
- 如果你使用了指针,确保指针不为空,且指向了有效的vector对象。
- 考虑使用智能指针等RAII机制来管理vector的生命周期,以避免手动管理引起的错误。
相关问题
line 1034: char 9: runtime error: reference binding to null pointer of type 'std::vector<int, std::allocator<int>>' (stl_vector.h) summary: undefinedbehaviorsanitizer: undefined-behavior /usr/bin/../lib/gcc/x86_64-linux-gnu/9/../../../../include/c++/9/bits/stl_vector.h:1043:9
### 回答1:
这是一个运行时错误,错误信息为“在类型为'std::vector<int, std::allocator<int>>'的空指针上进行引用绑定(stl_vector.h)”。这意味着某个变量被赋予了空值,在引用时导致程序崩溃。这是一个未定义的行为。
### 回答2:
这段错误信息实际上来自于一个C++程序的编译器,在运行时出现了一个运行错误,具体是在程序执行到第1034行时,出现了一个引用绑定到了一个空指针的异常错误。而这个指针的类型是一个整型的向量,使用了std::vector标准库来实现。
更具体地说,STL vector的构造函数有一个构造函数,其中传递的参数类型为std::initializer_list。在上述的错误信息中,STL vector库中的代码出现了一个引用到了空指针的情况,这种情况实际上是在程序运行时出现的,是一种未定义的行为。这种情况的出现是因为在程序中没有正确地初始化vector的指针,或者是在程序中涉及到了一些内存分配等相关问题导致的。最终导致的结果就是程序运行时出现异常,无法完成正常的执行。
因此,要解决这个问题,我们需要检查程序中关于STL vector的内容,尤其是在使用std::initializer_list类型参数时,需要确保这些参数都得到正确的初始化。另外,我们还可以考虑调试程序,检查程序在运行时时段的内存分配情况,以找到导致程序出现异常的具体原因,进行相应的修复。
### 回答3:
这个错误是由于引用空指针导致的运行时错误。在这个特定的程序中,错误发生在第1034行,第9个字符处,这个位置的代码正在尝试使用类型为'std :: vector <int,std :: allocator <int>>'的空指针进行引用绑定。这意味着代码试图将一个没有值的指针与另一个变量进行绑定,这样会导致运行时错误。
这个错误可能有很多种不同的原因,但通常是由于代码逻辑错误或使用不正确的内存分配方法所导致的问题。在处理这种类型的错误时,我们需要检查代码逻辑和内存管理是否有问题。如果我们可以找到这个问题的确切原因,我们就可以采取适当的措施来修复错误。
为了预防这种错误的发生,我们需要遵循正确的编程规范和内存管理技巧。我们需要确保我们的代码是有效的,并避免使用未初始化的变量。我们还需要确保我们使用了正确的内存分配方法,并确保释放了我们不再使用的内存。如果我们能够遵循这些规则,我们就可以大大减少程序错误和运行时错误的发生。
reference binding to null pointer of type 'std::vector<int, std::allocator<int>>' (stl_vector.h)
This error occurs when you try to reference a null pointer of type 'std::vector<int, std::allocator<int>>'. It means that you have a vector object that has not been properly initialized or has been deleted, and you are trying to access its elements.
To fix this error, you need to make sure that the vector object is properly initialized and not deleted before you access its elements. You can also check if the pointer is null before accessing its elements to avoid this error.
For example, the following code will result in the error:
```
std::vector<int> *v = nullptr;
int x = (*v)[0]; // error: reference binding to null pointer of type 'std::vector<int, std::allocator<int>>'
```
To fix this error, you need to initialize the vector object before accessing its elements:
```
std::vector<int> v = {1, 2, 3};
int x = v[0]; // no error
```
Or check if the pointer is null before accessing the elements:
```
std::vector<int> *v = nullptr;
if (v != nullptr) {
int x = (*v)[0]; // no error
}
```
阅读全文
相关推荐
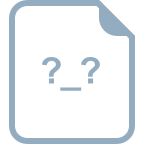
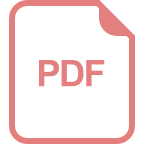
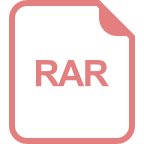



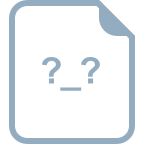
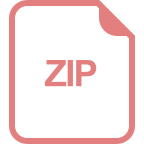
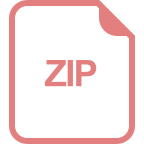
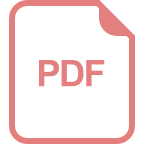
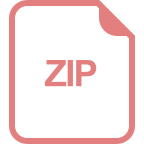
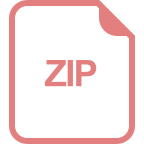
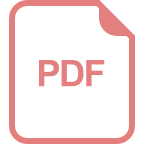
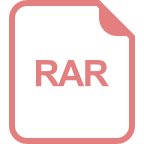
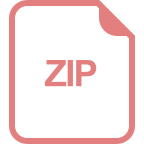
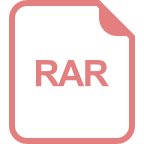
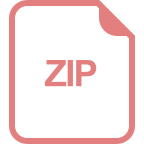