linux pthread_create 优先级
时间: 2023-11-15 19:56:52 浏览: 613
在Linux中,pthread_create函数创建线程时可以指定线程的优先级。可以使用pthread_attr_setschedparam函数设置线程的调度参数,其中包括线程的优先级。默认情况下,线程的优先级为0,最高优先级为99。可以使用sched_get_priority_max和sched_get_priority_min函数获取系统支持的最高和最低优先级。
相关问题
pthread_create指定核创建任务
在POSIX线程库(pthread)中,`pthread_create`函数用于在指定的线程上下文中创建一个新的线程。该函数的基本原型如下:
```c
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine)(void*), void *arg);
```
其中:
- `pthread_t *thread`:指向新创建线程标识符的指针,函数返回后将填充实际的线程ID。
- `const pthread_attr_t *attr`:指向`pthread_attr_t`结构体的指针,用于设置线程属性,如优先级、栈大小等,可以设为NULL表示使用默认属性。
- `void *(*start_routine)(void*)`:指向线程执行开始函数的指针,通常是一个函数指针,它接收一个`void *`类型的参数,并在线程内部进行处理。
- `void *arg`:作为`start_routine`的第一个参数传递给新线程。
如果你想让新线程在特定的CPU核心上运行,这通常是操作系统级别的操作,而不是通过`pthread_create`直接控制的。大多数现代系统并不允许用户直接指定线程绑定到特定的核心,因为调度策略是由操作系统自动管理的。不过,你可以尝试通过一些高级特性(如Linux的`SCHED_SETAFFINITY`)来间接影响线程的执行位置,但这需要对底层平台有深入理解。
pthread_create创建线程并绑定到硬核6的示例代码
在C语言中,`pthread_create`函数用于创建新的线程,并可以指定线程的优先级、堆栈大小等参数。如果想要创建一个线程并且将其绑定到特定的CPU核心(比如第6核),这在现代多核处理器上通常不可直接通过`pthread_create`实现,因为操作系统默认的线程调度策略通常是动态的,难以直接绑定。
但是,你可以使用Linux提供的`sched_setaffinity`系统调用来设置线程的亲缘性集合(Sibling Affinity),该集合指定了线程愿意运行在其兄弟(在同一CPU组内的其他线程)旁边。以下是一个简单的例子:
```c
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
#include <sched.h>
void *thread_function(void *arg) {
// 线程主函数
printf("Thread running on CPU %d\n", sched_getcpu());
// 这里假设已经设置了线程的亲缘性集合
return NULL;
}
int main() {
pthread_t thread_id;
cpu_set_t cpuset;
// 初始化cpuset,这里仅包含CPU 6
CPU_ZERO(&cpuset);
CPU_SET(5, &cpuset); // 注意,索引从0开始,所以5对应CPU 6
if (pthread_create(&thread_id, NULL, thread_function, NULL)) {
perror("Failed to create thread");
return -1;
}
// 尝试将线程绑定到cpuset
if (pthread_setaffinity_np(thread_id, sizeof(cpuset), &cpuset) != 0) {
perror("Failed to set affinity");
pthread_cancel(thread_id); // 如果失败,取消线程
return -1;
}
// 等待线程结束
pthread_join(thread_id, NULL);
return 0;
}
```
注意:实际操作中,能否成功将线程绑定到特定核心取决于系统的线程调度策略以及是否有足够的上下文切换开销。另外,在某些平台上,这种硬编码的CPU绑定可能会受限或者不可行。
阅读全文
相关推荐
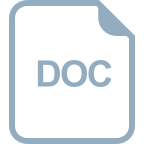
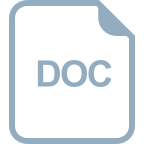
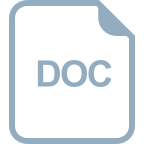
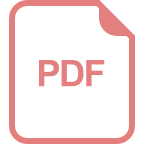
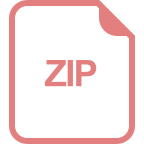
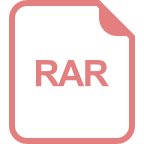
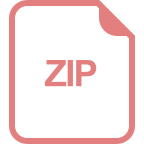
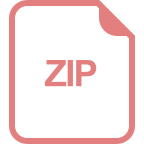
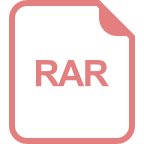
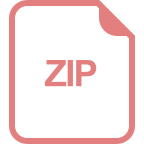
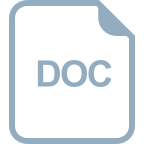
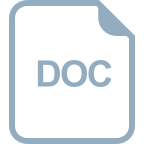
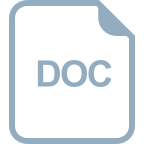

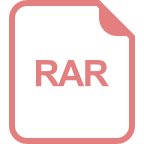
