springboot 配置websocket的完整方法 代码详细说明
时间: 2023-12-20 13:03:34 浏览: 76
当使用Spring Boot配置WebSocket时,可以按照以下步骤进行操作:
1. 添加依赖项:在`pom.xml`文件中添加以下依赖项,以使用Spring Boot的WebSocket支持:
```xml
<dependencies>
<!-- 其他依赖项 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
</dependencies>
```
2. 创建WebSocket配置类:创建一个配置类,用于配置WebSocket相关的Bean和路径。例如,创建一个名为`WebSocketConfig`的类,并添加`@Configuration`注解。
```java
import org.springframework.context.annotation.Configuration;
import org.springframework.web.socket.config.annotation.EnableWebSocket;
import org.springframework.web.socket.config.annotation.WebSocketConfigurer;
import org.springframework.web.socket.config.annotation.WebSocketHandlerRegistry;
@Configuration
@EnableWebSocket
public class WebSocketConfig implements WebSocketConfigurer {
@Override
public void registerWebSocketHandlers(WebSocketHandlerRegistry registry) {
registry.addHandler(new YourWebSocketHandler(), "/your-websocket-endpoint")
.setAllowedOrigins("*");
}
}
```
3. 创建WebSocket处理程序:创建一个处理程序类,用于处理WebSocket连接和消息。例如,创建一个名为`YourWebSocketHandler`的类,并实现`WebSocketHandler`接口。
```java
import org.springframework.web.socket.TextMessage;
import org.springframework.web.socket.WebSocketSession;
import org.springframework.web.socket.handler.TextWebSocketHandler;
public class YourWebSocketHandler extends TextWebSocketHandler {
@Override
public void afterConnectionEstablished(WebSocketSession session) throws Exception {
// 在建立WebSocket连接时执行的逻辑
}
@Override
protected void handleTextMessage(WebSocketSession session, TextMessage message) throws Exception {
// 处理接收到的文本消息
}
}
```
4. 运行Spring Boot应用程序:运行你的Spring Boot应用程序,并确保WebSocket配置和处理程序已经生效。
现在,你可以使用WebSocket客户端连接到`/your-websocket-endpoint`路径,并与服务器进行双向通信。
希望以上信息能帮助到你。如果有任何进一步的问题,请随时提问。
阅读全文
相关推荐
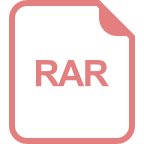
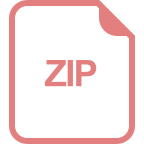
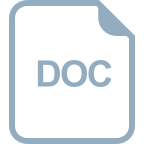






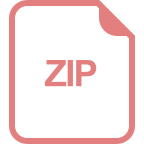
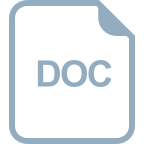





