c++ 怎么唤醒sleep的线程
时间: 2024-05-17 20:13:42 浏览: 16
在C++中,可以使用std::condition_variable来唤醒一个正在等待的线程。具体的操作可以分为两个步骤:
1. 在等待线程中调用wait()函数,将线程挂起。
2. 在唤醒线程中调用notify_one()或者notify_all()函数,通知等待线程继续执行。
下面是一个简单的示例代码:
```c++
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
std::mutex mtx;
std::condition_variable cv;
bool ready = false;
void worker_thread()
{
// 等待main线程通知
std::unique_lock<std::mutex> lock(mtx);
while (!ready) {
cv.wait(lock);
}
// 线程被唤醒,继续执行
std::cout << "Worker thread is running!" << std::endl;
}
int main()
{
// 启动worker线程
std::thread worker(worker_thread);
// 做一些其他的事情
std::cout << "Main thread is running!" << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
// 通知worker线程继续执行
{
std::lock_guard<std::mutex> lock(mtx);
ready = true;
}
cv.notify_one();
// 等待worker线程执行完毕
worker.join();
return 0;
}
```
在上面的代码中,worker线程在等待main线程通知后才会继续执行。在main线程中,我们通过改变ready的值和调用notify_one()函数来通知worker线程继续执行。
相关推荐
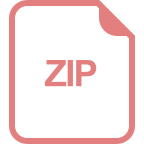
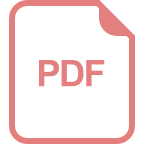
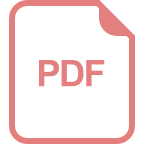














