用c++设计一个类解决约瑟夫环
时间: 2024-04-29 10:22:05 浏览: 107
以下是使用 C++ 设计的一个类,用于解决约瑟夫环问题:
```cpp
#include <iostream>
#include <list>
class Josephus {
public:
Josephus(int n, int m) : n_(n), m_(m) {}
// 执行约瑟夫环算法
void run() {
// 初始化链表
for (int i = 0; i < n_; ++i) {
people_.push_back(i);
}
// 开始执行约瑟夫环
auto it = people_.begin();
while (n_ > 1) {
// 找到要删除的人的位置
for (int i = 1; i < m_; ++i) {
++it;
if (it == people_.end()) {
it = people_.begin();
}
}
// 删除该位置上的人
it = people_.erase(it);
if (it == people_.end()) {
it = people_.begin();
}
// 更新当前链表长度
--n_;
}
// 输出最后剩下的人
std::cout << "The last person is: " << people_.front() << std::endl;
}
private:
int n_; // 约瑟夫环的人数
int m_; // 每次报数的数字
std::list<int> people_; // 链表,存储当前还在圈内的人
};
int main() {
// 假设有10个人,每次数到3就出圈,求最后留下的人
Josephus josephus(10, 3);
josephus.run();
return 0;
}
```
在上述代码中,我们使用 STL 中的 `list` 容器来实现约瑟夫环。具体来说,每次找到要删除的人的位置时,我们使用迭代器 `list<int>::iterator` 来指向链表中的对应位置,并使用 `erase` 函数来将该位置上的元素删除。需要注意的是,删除元素后,迭代器 `it` 需要指向删除元素的下一个位置,否则会造成指针错误。
阅读全文
相关推荐




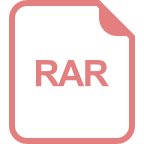




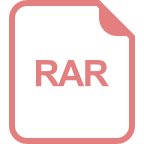


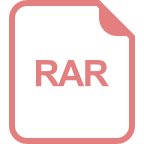




