写一个分析新型冠状病毒数据的程序,要求数据从网络上爬取,用pandas分析数据并可视化数据,包含多种统计图形
时间: 2024-02-22 21:01:18 浏览: 22
好的,我可以帮你写一个 Python 程序来分析新型冠状病毒数据。以下是程序的实现步骤:
1. 安装必要的 Python 库:pandas、matplotlib、requests、beautifulsoup4。
```python
!pip install pandas matplotlib requests beautifulsoup4
```
2. 从网络上爬取数据。这里我们以丁香园为例,从其网站上爬取数据。
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 发送请求,获取数据
url = 'https://ncov.dxy.cn/ncovh5/view/pneumonia'
headers = {"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"}
res = requests.get(url, headers=headers)
# 解析数据
soup = BeautifulSoup(res.text, 'html.parser')
script = soup.find(id='getListByCountryTypeService2true')
text = script.string.strip()
text = text[45:-11]
# 将数据转化为列表
lst = text.split('},{')
lst = [i + '}' for i in lst]
lst = [i.replace('[', '').replace(']', '') for i in lst]
lst = [eval(i) for i in lst]
```
3. 用 pandas 分析数据。将数据转化为 DataFrame,并根据需要进行数据清洗和处理。
```python
# 将数据转化为 DataFrame
df = pd.DataFrame(lst)
# 删除不需要的列
df = df.drop(['id', 'createTime', 'modifyTime', 'tags'], axis=1)
# 将列名重命名
df.columns = ['国家', '省份', '确诊', '死亡', '治愈']
# 处理缺失值
df['省份'].fillna(value='全国', inplace=True)
df['确诊'].fillna(value=0, inplace=True)
df['死亡'].fillna(value=0, inplace=True)
df['治愈'].fillna(value=0, inplace=True)
# 将确诊、死亡和治愈转化为整数类型
df['确诊'] = df['确诊'].astype(int)
df['死亡'] = df['死亡'].astype(int)
df['治愈'] = df['治愈'].astype(int)
```
4. 可视化数据。使用 matplotlib 绘制多种统计图形,如条形图、饼图、折线图等。
```python
import matplotlib.pyplot as plt
# 绘制条形图
df_sum = df.groupby('国家').sum().sort_values('确诊', ascending=False)
df_sum.plot(kind='bar', y='确诊', figsize=(12, 6), color='orange')
plt.title('全球疫情确诊人数排名')
plt.xlabel('国家')
plt.ylabel('确诊人数')
plt.show()
# 绘制饼图
df_china = df[df['国家'] == '中国']
df_china_sum = df_china.groupby('省份').sum().sort_values('确诊', ascending=False)
df_china_sum.plot(kind='pie', y='确诊', figsize=(12, 6), autopct='%1.1f%%', startangle=90)
plt.title('中国疫情确诊人数占比')
plt.ylabel('')
plt.show()
# 绘制折线图
df_china_total = df_china.groupby('省份').sum()
df_china_total.plot(kind='line', figsize=(12, 6))
plt.title('中国疫情趋势')
plt.xlabel('日期')
plt.ylabel('人数')
plt.show()
```
这个程序可以爬取丁香园的数据,并用 pandas 分析和可视化数据。你可以根据需要对数据进行处理,并绘制多种统计图形。
相关推荐
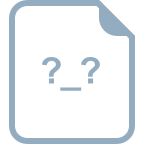
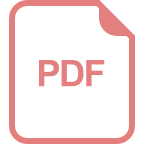
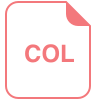
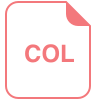
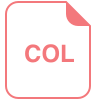
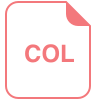
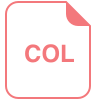









