uint16_t MB_CRC16(uint8_t *pFrame, uint16_t count) { uint8_t CRCHi = 0xFF; uint8_t CRCLo = 0xFF; int32_t index; while(count‐‐) { index = CRCLo ^ *(pFrame++); CRCLo = (uint8_t)(CRCHi ^ _CRCHi[index]); CRCHi = _CRCLo[index]; } return (uint16_t)(CRCHi << 8 | CRCLo ); }
时间: 2024-04-20 17:26:54 浏览: 108
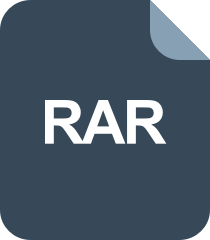
简单的CRC16计算器

这段代码实现了一个基于CRC16校验算法的函数,用于计算输入数据的校验值。函数的参数是一个指向输入数据缓冲区的指针和输入数据的长度。函数返回一个16位的校验值。该函数使用了一个名为_CRCHi和一个名为_CRCLo的查找表,其中_CRCHi是CRC高位查找表,_CRCLo是CRC低位查找表。
函数的实现过程是:首先初始化CRCHi和CRCLo为0xFF;然后对输入数据进行遍历,每次取出一个字节并与CRCLo进行异或运算,得到一个索引值;然后使用_CRCHi和_CRCLo查找表中的对应值更新CRCHi和CRCLo的值;最后返回计算得到的校验值。
注意:该函数并没有进行输入数据的合法性验证,因此在使用时需要确保输入数据的正确性。
阅读全文
相关推荐
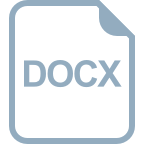
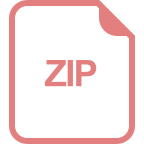



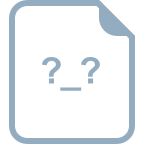
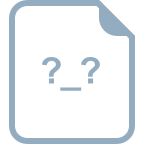
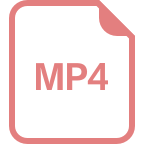