C++ 怎么将map使用std::sort储存
时间: 2023-10-24 13:12:08 浏览: 150
要使用std::sort对map进行排序,您需要将map的元素存储在一个vector中,然后对该vector进行排序。以下是一个示例代码:
```c++
#include <iostream>
#include <map>
#include <vector>
#include <algorithm>
int main()
{
std::map<int, std::string> myMap = {{3, "C"}, {1, "A"}, {2, "B"}};
std::vector<std::pair<int, std::string>> vec(myMap.begin(), myMap.end());
std::sort(vec.begin(), vec.end());
for (const auto& p : vec)
{
std::cout << p.first << " " << p.second << std::endl;
}
return 0;
}
```
在这个示例中,我们首先创建了一个map对象myMap,其中包含三个元素。然后,我们使用begin()和end()函数将myMap的元素复制到一个名为vec的vector中。最后,我们使用std::sort对vec进行排序,使用for循环遍历vec并输出每个元素的值。请注意,在vec中,每个元素都是一个std::pair,其中第一个元素是int类型的键,第二个元素是std::string类型的值。
相关问题
c++中使用std::sort对十一个整型变量进行排序,并获取排序后每个变量对应的变量名
在C++中,如果你想对一组整型变量进行排序并同时保持它们的原始顺序,通常我们会先创建一个结构体(struct)或者关联数组(如pair),然后将变量名和数值作为这个结构体的成员。这里假设我们有一个名为`Variable`的简单结构体,包含一个int类型的值和一个std::string类型的变量名:
```cpp
#include <iostream>
#include <vector>
#include <map>
#include <algorithm> // 包含sort函数
// 定义一个结构体,用于存储整数和变量名
struct Variable {
int value;
std::string name;
};
// 比较函数,用于sort函数
bool compareVariables(const Variable& a, const Variable& b) {
return a.value < b.value; // 按照值从小到大排序
}
int main() {
std::vector<Variable> variables = {{"x", 5}, {"y", 9}, {"z", 1}, {"a", 7}, ...}; // 十一个整型变量及其名字
std::sort(variables.begin(), variables.end(), compareVariables); // 对vector按值排序
for (const auto& var : variables) {
std::cout << "Sorted variable " << var.name << " has a value of " << var.value << "\n";
}
// 获取排序后的变量名,但注意变量名不会改变,只是按照值排序了
for (size_t i = 0; i < variables.size(); ++i) {
std::cout << "Original name for position " << i << ": " << variables[i].name << "\n";
}
return 0;
}
```
在这个例子中,`std::sort`会改变`variables`向量中元素的顺序,但每个`Variable`对象的`name`字段并没有实际改变,因为它们的值仍然是原来的。
std::sort 排序 std::map
`std::sort`是C++标准库中的一个函数模板,主要用于对容器中的元素进行排序。然而,它不能直接用来排序`std::map`。原因在于`std::map`本身是一种基于键值对的容器,并且它默认按照键的顺序存储元素,也就是说`std::map`内部就是有序的。`std::map`的排序规则是基于其键的比较函数`std::less`,或者是用户定义的比较函数。
如果要使用`std::sort`来对`std::map`中的元素进行排序,你需要先将`std::map`中的元素复制到一个可以排序的容器中,比如`std::vector`,然后使用`std::sort`对这个容器进行排序。排序后,你可以将排序后的元素再复制回`std::map`(如果需要的话),但这样做通常没有太大的实际意义,因为`std::map`的有序特性在操作完成后可能会被破坏。
如果你想要在遍历`std::map`时按照特定的顺序输出元素,可以通过对`std::map`的迭代器进行排序来实现。例如,如果你想根据键的值进行升序排序,可以直接使用默认的`std::less`比较函数:
```cpp
#include <iostream>
#include <map>
#include <vector>
#include <algorithm> // for std::sort
int main() {
std::map<int, int> m = {{3, 1}, {1, 2}, {2, 3}};
// 将map的键值对复制到vector中
std::vector<std::pair<int, int>> v(m.begin(), m.end());
// 使用默认的比较函数std::less对vector进行排序
std::sort(v.begin(), v.end());
// 输出排序后的元素
for (const auto& p : v) {
std::cout << p.first << ": " << p.second << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
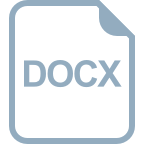
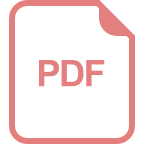
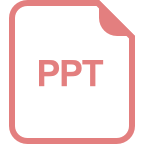
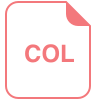
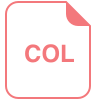
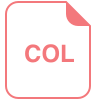
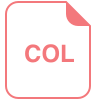
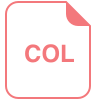
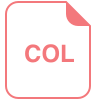
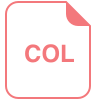
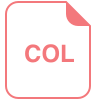
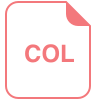
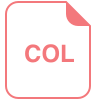


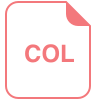
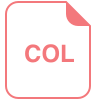